Okay, so today I wanted to mess around with something I’ve been calling “state rings.” The basic idea is to cycle through a set of states in a, well, ring-like fashion. Think of it like a light switch with multiple settings, or maybe different modes for a game character. I’ve played with this concept before but never really nailed down a clean, reusable way to do it.
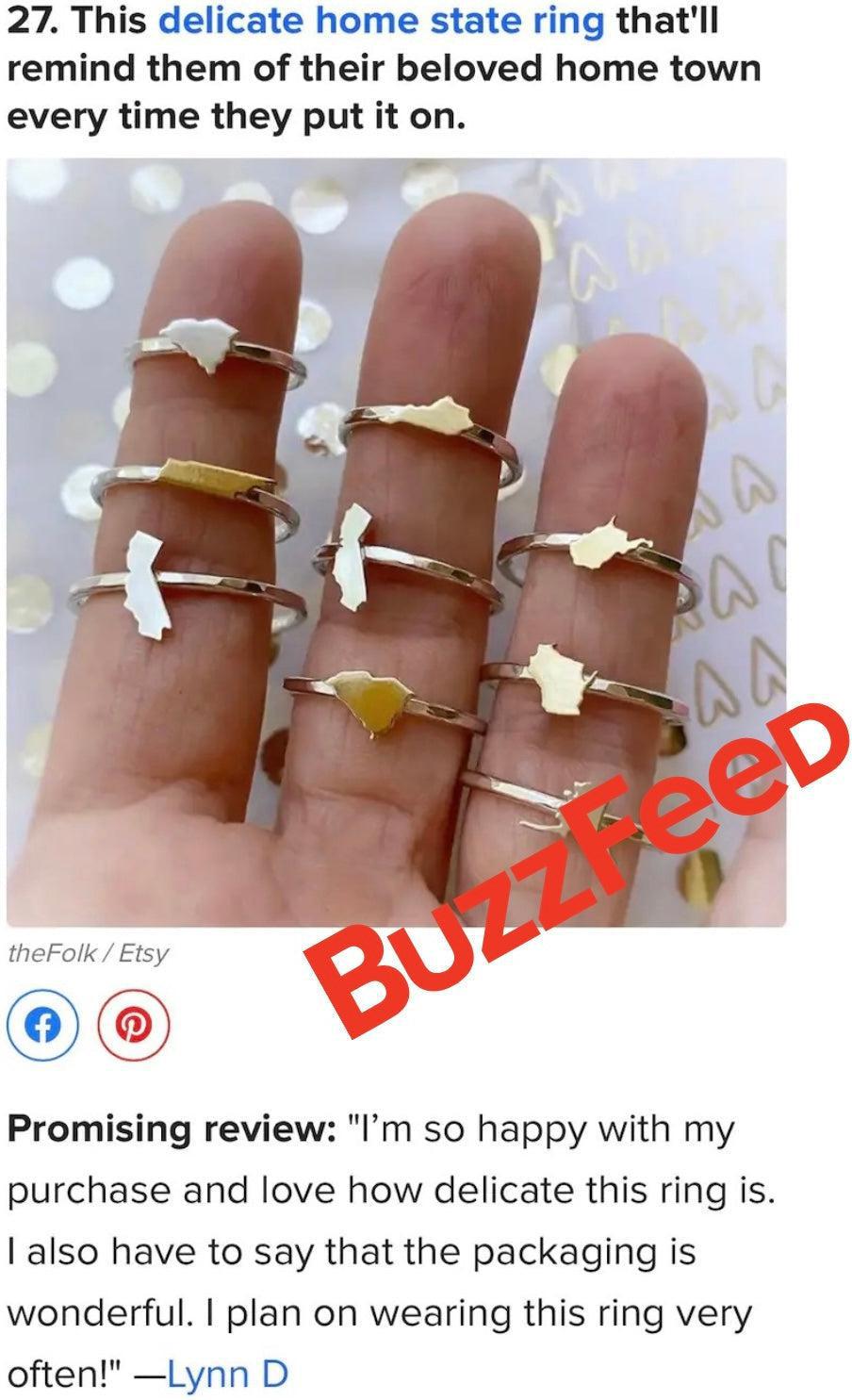
First, I doodled some notes on paper. Just trying to visualize how the data would flow. I figured I needed a way to store the current state, a way to define all possible states, and a way to move to the next state. Simple enough, right?
Then I fired up a new project. I decided to use a simple array to hold my states. Let’s say I have states like “idle,” “walking,” “running,” and “jumping.” I just threw those into an array.
Here are the basic state lists:
- idle
- walking
- running
- jumping
Next, I needed a variable to track the current state. I went with an index, starting at 0, which would point to “idle” in my array. So far, so good.
The tricky part was the cycling. I wanted a function that would just bump the index to the next state. But, I also needed it to wrap around. When I’m at “jumping” (the last state), the next state should be “idle” (the first state). That’s where the “ring” part comes in.
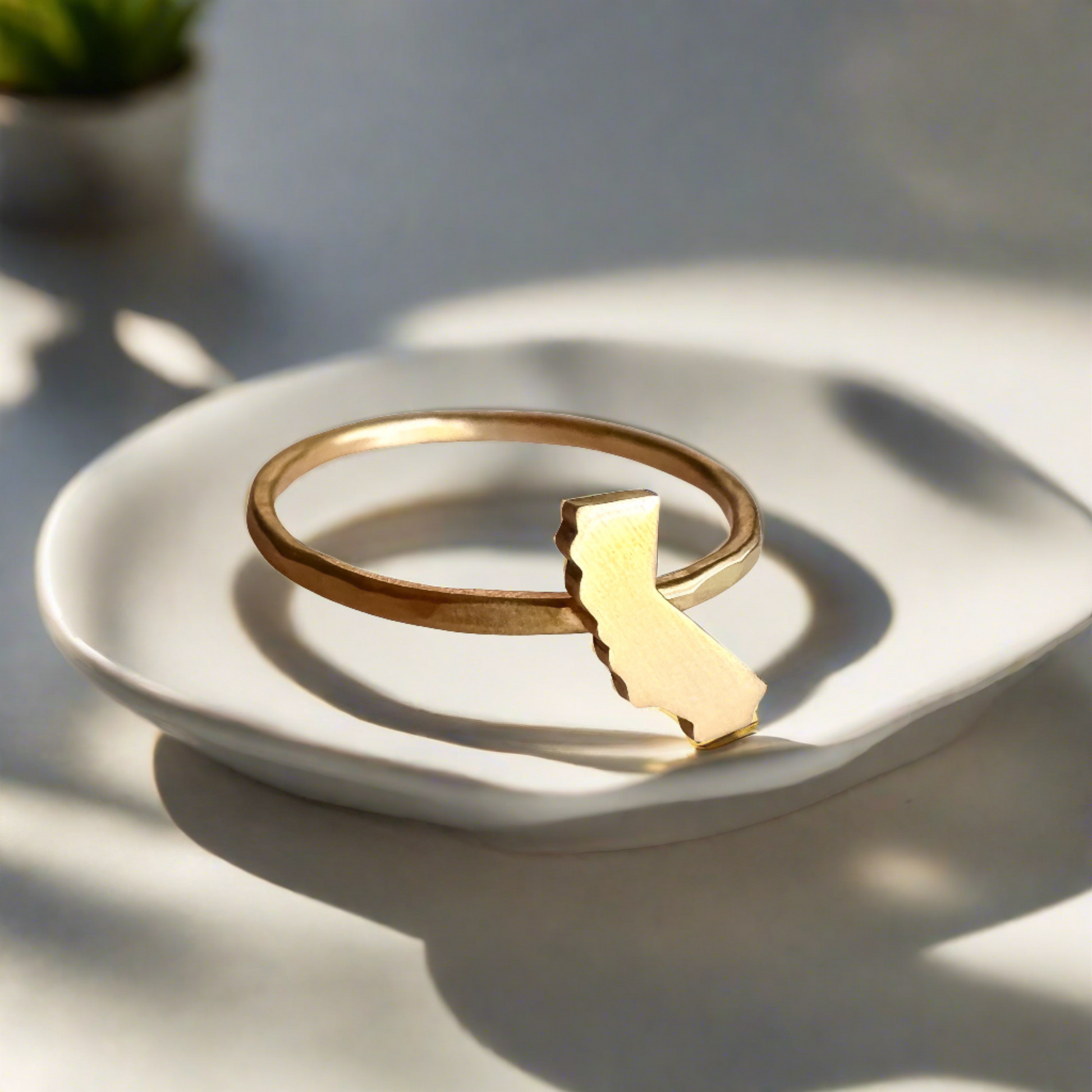
So, I whipped up a little function. It basically took the current index, added 1 to it, and then used the modulo operator (%) with the length of the state array. That modulo is the key! It gives you the remainder after division. So, if my index is 3 (pointing to “jumping”) and I add 1, I get 4. But 4 % 4 (the length of the array) is 0. Boom! Back to “idle”.
I tested this out a bunch, just printing the current state each time I cycled. It worked like a charm! Each time I called my function, it moved to the next state, and when it hit the end, it smoothly looped back to the beginning.
Finally, I wrapped everything up in a neat little class. Now I can just create a new “state ring” whenever I need one, give it a list of states, and cycle through them with a single function call. It’s super clean and reusable, which is exactly what I was aiming for.
It is really cool!