Okay, so today I’m gonna walk you through how I tackled that “division for a tennis match nyt” thing. Saw it floating around and thought, “Hey, why not give it a shot?” It turned out to be a bit trickier than I initially thought, but hey, that’s why we practice, right?
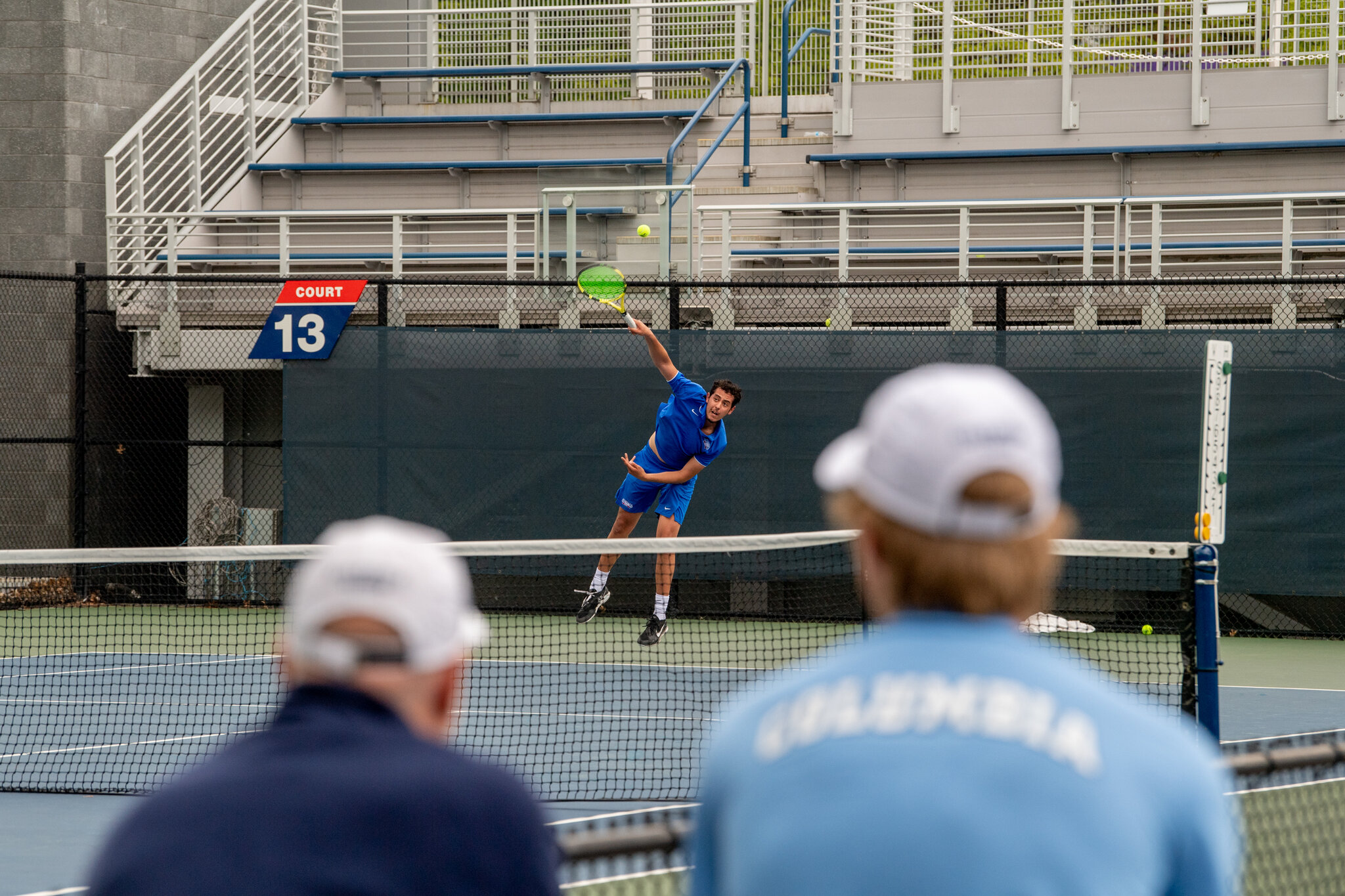
First off, I had to really sit down and understand what the heck the question was even asking. It wasn’t just straight-up division; it was about figuring out how to split players fairly for a tennis match, probably with some constraints or conditions. I started by jotting down all the possible scenarios and constraints I could think of.
Then, I decided to use Python because, well, it’s my go-to language for pretty much everything. I started with a really basic function that took the number of players as input. The first version just tried to divide the players into two equal teams. Something super simple like:
python
def divide_players(num_players):
if num_players % 2 == 0:
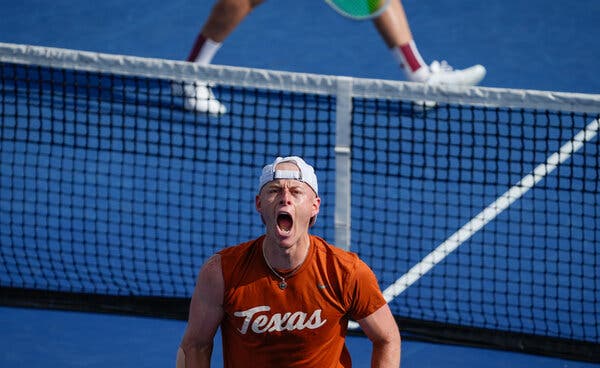
team_size = num_players // 2
return team_size, team_size
else:
return None # or some other handling
But, of course, the real challenge was more complex. The problem probably involved some sort of skill level consideration or some other hidden condition. So, I started thinking about how to incorporate that. I tried a few different approaches. First, I looked into using some basic sorting algorithms to group players based on assumed skill levels (even if I had to make up those skill levels for testing purposes).
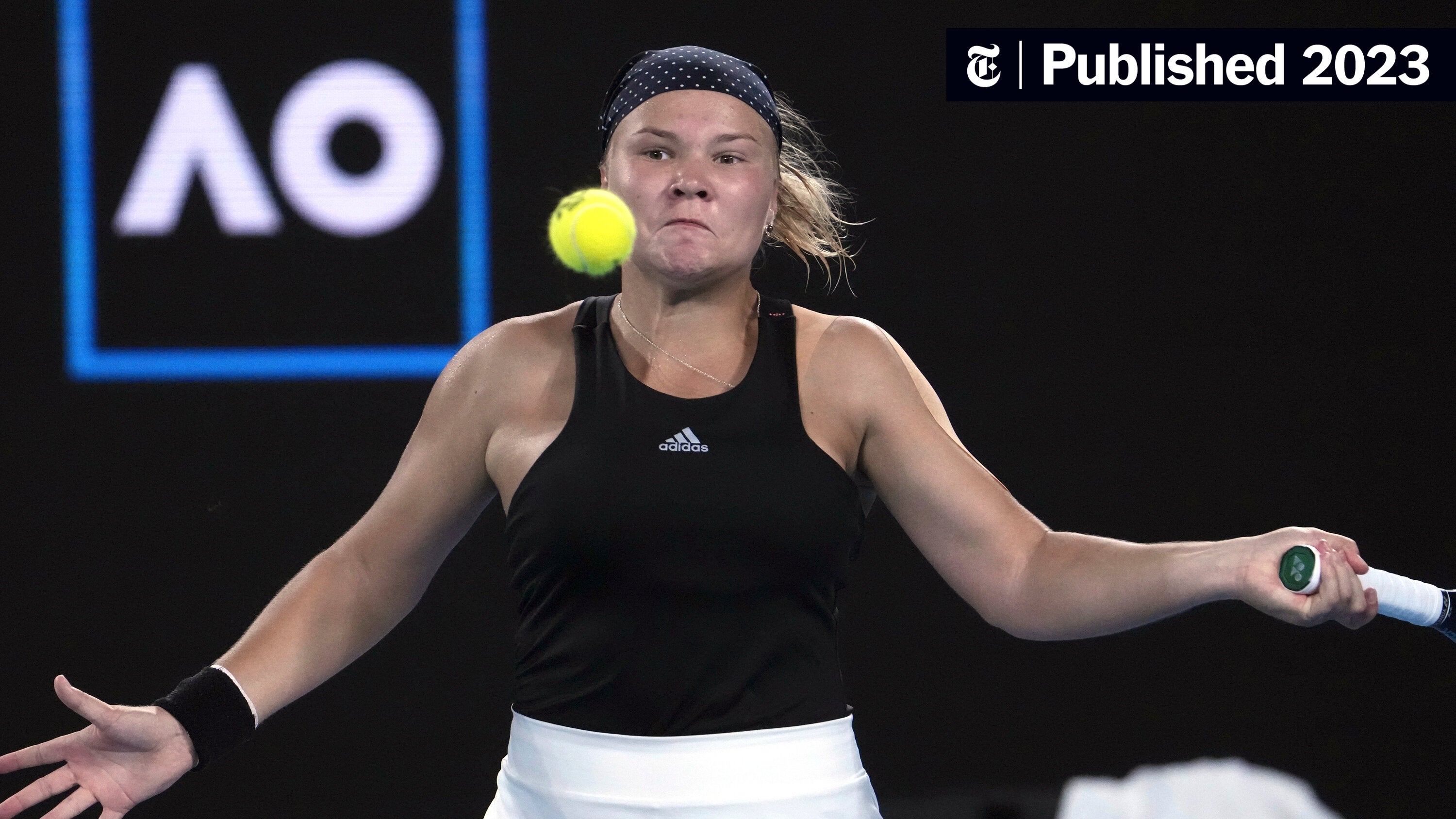
I spent a good chunk of time experimenting with different sorting methods. Quick sort, merge sort, just to see which one would give me the best performance. Turns out, for a reasonably small number of players, it didn’t really matter that much.
The real breakthrough came when I realized I needed to think about the problem in terms of minimizing the difference in the sum of skill levels between the two teams. That’s when I started to explore dynamic programming. It’s a bit overkill for a tennis match division, but hey, it’s good practice!
I coded up a dynamic programming solution that tried all possible combinations of players to find the split that minimized the difference in skill level sums. It was definitely more complicated, involving creating a table to store intermediate results and backtracking to find the optimal solution.
Here’s a rough sketch of what the dynamic programming approach looked like (in simplified terms):
- Create a table to store whether a specific sum of skill levels can be achieved with a subset of players.
- Iterate through the players and update the table.
- Find the sum closest to half the total skill level sum.
- Backtrack to determine which players belong to which team.
It was a headache to debug, and I spent a lot of time staring at the code, adding print statements everywhere, and stepping through it with a debugger. But eventually, I got it working!
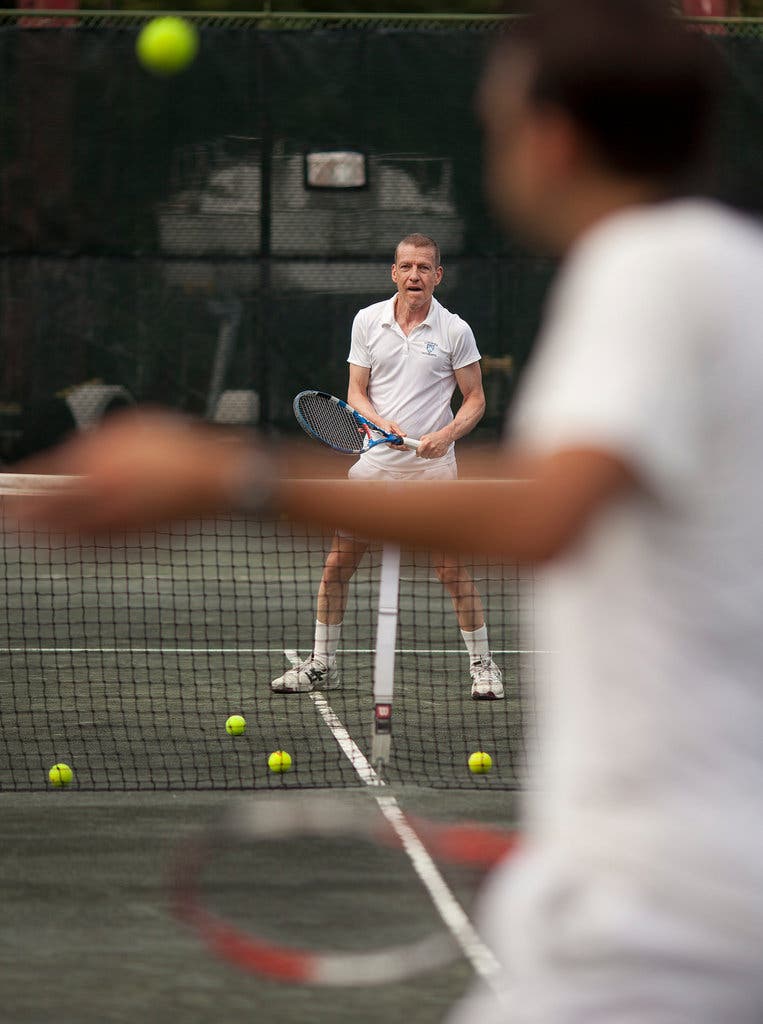
Finally, I tested it with various inputs, different numbers of players, and different skill level distributions. It wasn’t perfect (dynamic programming can get slow with a large number of players), but it gave a pretty good, fair division. And I learned a ton about dynamic programming along the way!
So, that’s basically how I approached the “division for a tennis match nyt” problem. It was a fun little exercise that pushed me to think about algorithm design and optimization. Maybe there’s a better way to do it, but that’s what I came up with!