Okay, so today I’m gonna walk you through how I tackled this “meet and greet word ladder answer key” thing. It was kinda fun, actually, like a little puzzle.
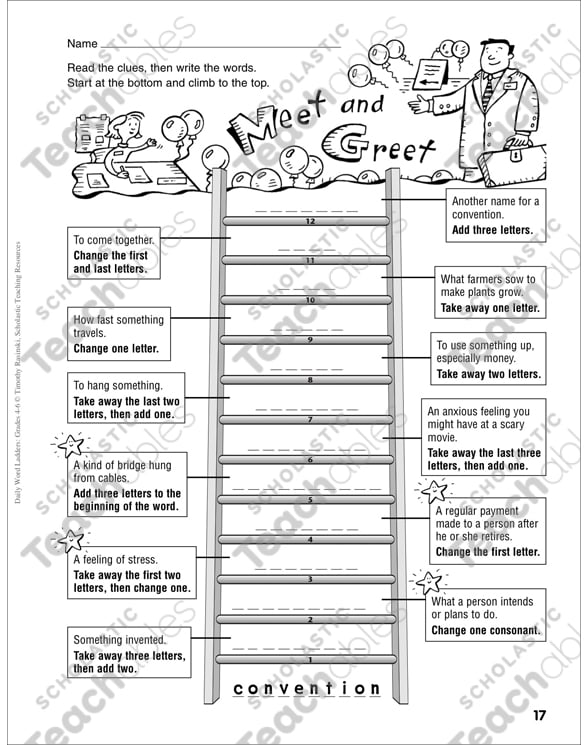
First off, I Googled that phrase, right? Just to see what’s already out there. I saw a bunch of worksheets and stuff, but nothing that really solved it for me in a programmatic way, which is what I was after.
So, I decided to just dive in. The basic idea of a word ladder is you start with one word and change one letter at a time to get to another word, and each step has to be a valid word.
I started by thinking about how to represent the words. I figured a list of strings in Python would be the easiest. Then, I needed a word list to check if each new word was valid. I grabbed a pretty big word list text file off the internet. Nothing fancy, just a plain text file with one word per line.
Next up, the core logic. I thought, “Okay, how do I find words that are one letter different?” I wrote a function that takes a word and the word list, and spits out all the words in the word list that are exactly one letter different. It just iterates through the word list and compares each word to the input word. If they’re the same length, it counts the different letters. If there’s only one difference, it adds it to the result list.
That was the slow part. Comparing every word to every other word is not super efficient. But hey, it worked for the size of word lists I was playing with.
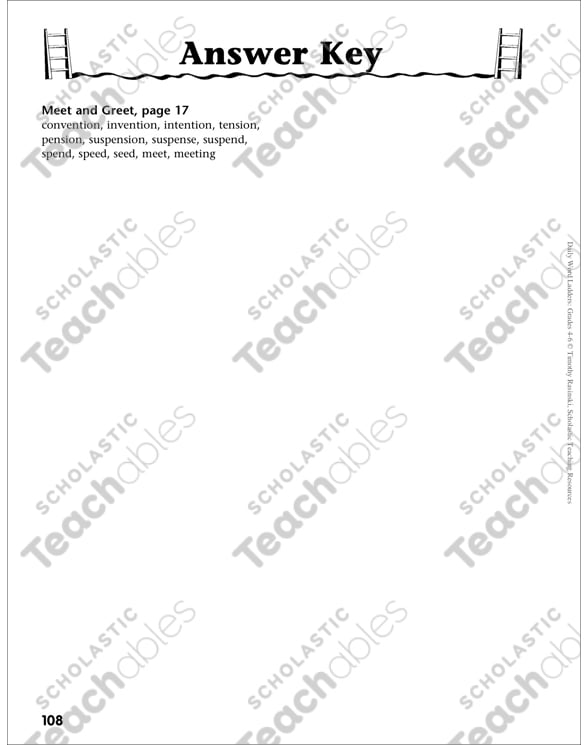
Then came the fun part – actually building the ladder. I used a Breadth-First Search (BFS). I know, sounds fancy, but it’s really just exploring all the possibilities level by level.
- I started with the ‘meet’ word
- I found all the one-letter-different words.
- Then, for each of those words, I found their one-letter-different words.
- I kept doing that until I hit ‘greet’.
To keep track of everything, I used a queue. Each item in the queue was a list of words – the current “ladder” I was building. If the last word in the ladder was ‘greet’, I was done! If not, I grabbed the last word, found all its neighbors, and added new ladders to the queue for each neighbor.
A really important thing was making sure I didn’t revisit words. Otherwise, I’d get stuck in infinite loops. So, I kept a set of “visited” words. Before adding a new ladder to the queue, I checked if the last word in it was already in the visited set. If it was, I skipped it.
It took a little tweaking to get it right. I had some off-by-one errors in my letter-comparison code, and I definitely had some infinite loop problems at first because I forgot to mark words as visited. But after some debugging, it worked! I got a ladder from “meet” to “greet”.
So, yeah, that’s pretty much it. Grabbed a word list, wrote a one-letter-difference function, used BFS to build the ladder, and made sure to avoid loops. It’s not the most elegant code in the world, but it got the job done, and it was a good little coding challenge.
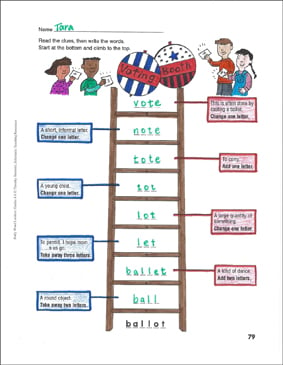
I mean, I could probably optimize it by using a better data structure for the word list, like a trie, or by using a more efficient algorithm for finding one-letter-different words, but for a quick and dirty solution, this was good enough.
And hey, I learned a thing or two along the way. Always a win!