So, I decided to mess around with this “consolation game” idea. I’d been seeing it pop up in a few places, and it sounded like a fun little project to kill some time. I figured I’d share my process, bumps and all, in case anyone else is curious about giving it a shot.
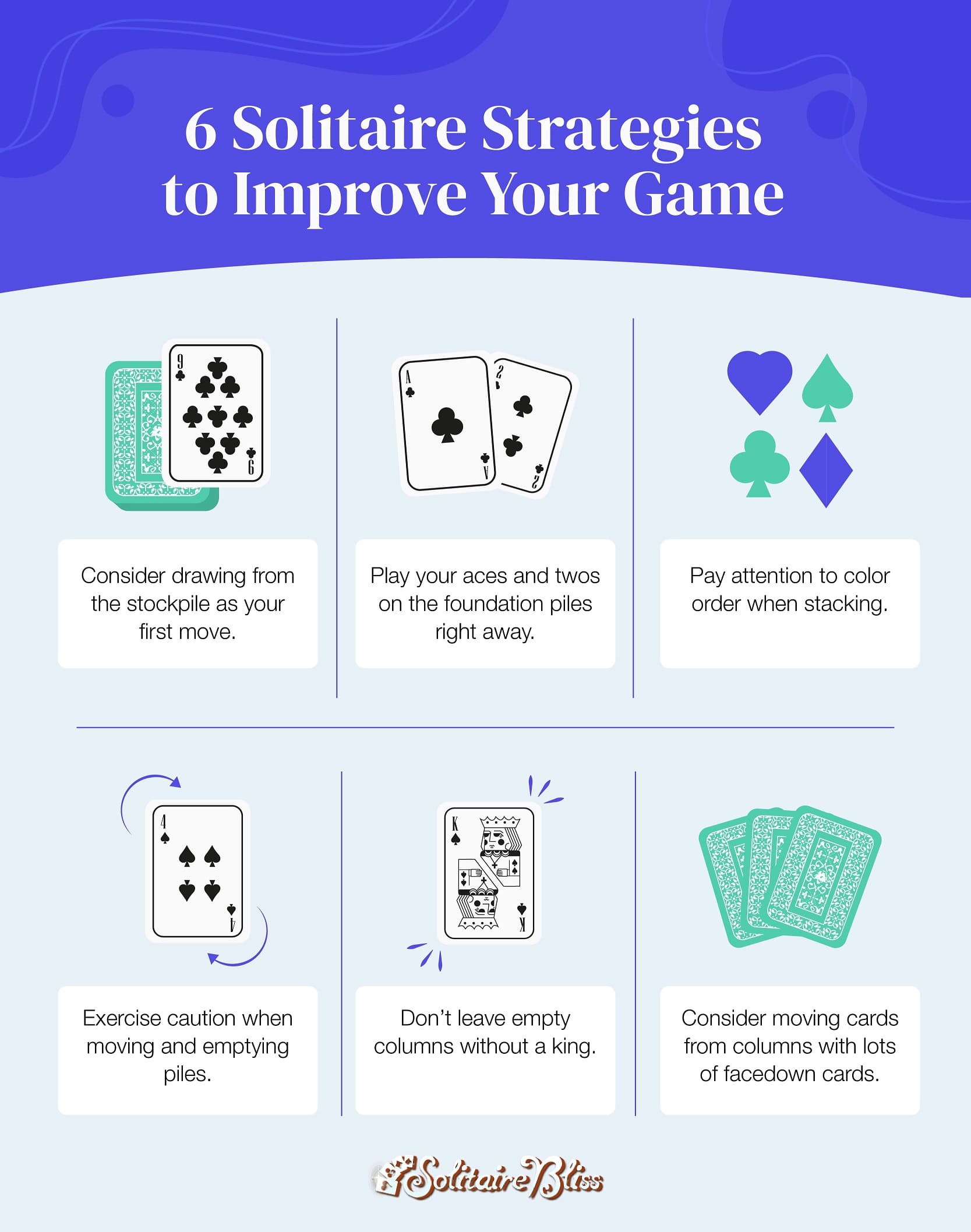
Getting Started
First things first, I needed to figure out what the heck a “consolation game” even was. Turns out, it’s basically a simple game where you try to make the best of a bad situation, digitally speaking. I wanted something I could whip up quickly, so I went with a super basic concept: a falling blocks game, but instead of trying to clear lines, you’re trying to avoid clearing them. The more cluttered your screen gets, the better you’re doing.
I opted to use plain old JavaScript, HTML, and CSS. No fancy frameworks or anything, just keeping it old-school. I started by setting up the basic HTML structure:
- Created a
<div>
to act as the game container. - Added a
<canvas>
element inside that<div>
, which is where the actual game would be drawn. - Threw in a
<p>
tag to display the “score” (which, remember, is actually how badly you’re doing).
Then, in my CSS, I did some super basic styling. Just gave the game container a border, set the canvas size, and made sure everything was centered. Nothing pretty, just functional.
The JavaScript Shenanigans
Now for the fun part: the JavaScript. This is where the game actually comes to life. I broke it down into a few key parts:
- Block Creation: I made a function to generate random block shapes. I went with the classic Tetris-style blocks, just because they’re easy to work with. Each block is just an array of coordinates, representing where the little squares that make up the block should be drawn.
- Game Loop: This is the heart of the game. I used
requestAnimationFrame
to create a loop that runs constantly. On each iteration, it does the following:- Clears the canvas.
- Moves the current falling block down a bit.
- Checks for collisions (with the bottom of the screen or other blocks).
- If there’s a collision, the block “settles” and a new one starts falling.
- Redraws everything (the settled blocks and the falling block).
- Updates the “score” (the number of settled blocks).
- Input Handling: I added event listeners to handle keyboard input. Left and right arrow keys move the block sideways, and the down arrow key makes it fall faster. Basic stuff.
- Collision Detection: This was the trickiest part, honestly. I had to write a function to check if the current falling block was overlapping with any of the settled blocks or going out of bounds. If it was, it meant the block couldn’t move any further.
Tweaking and Polishing
Once I had the basic game working, I spent some time tweaking things. I adjusted the falling speed, the block colors, and the size of the game board until I was happy with it. It’s still super simple, but it’s surprisingly addictive (or maybe I’m just easily amused). I could add more blocks, change the rules, or even add a “game over” condition if I wanted to, but for now, I’m calling it good. The key was to build something from a simple beginning, and keep adding step by step.
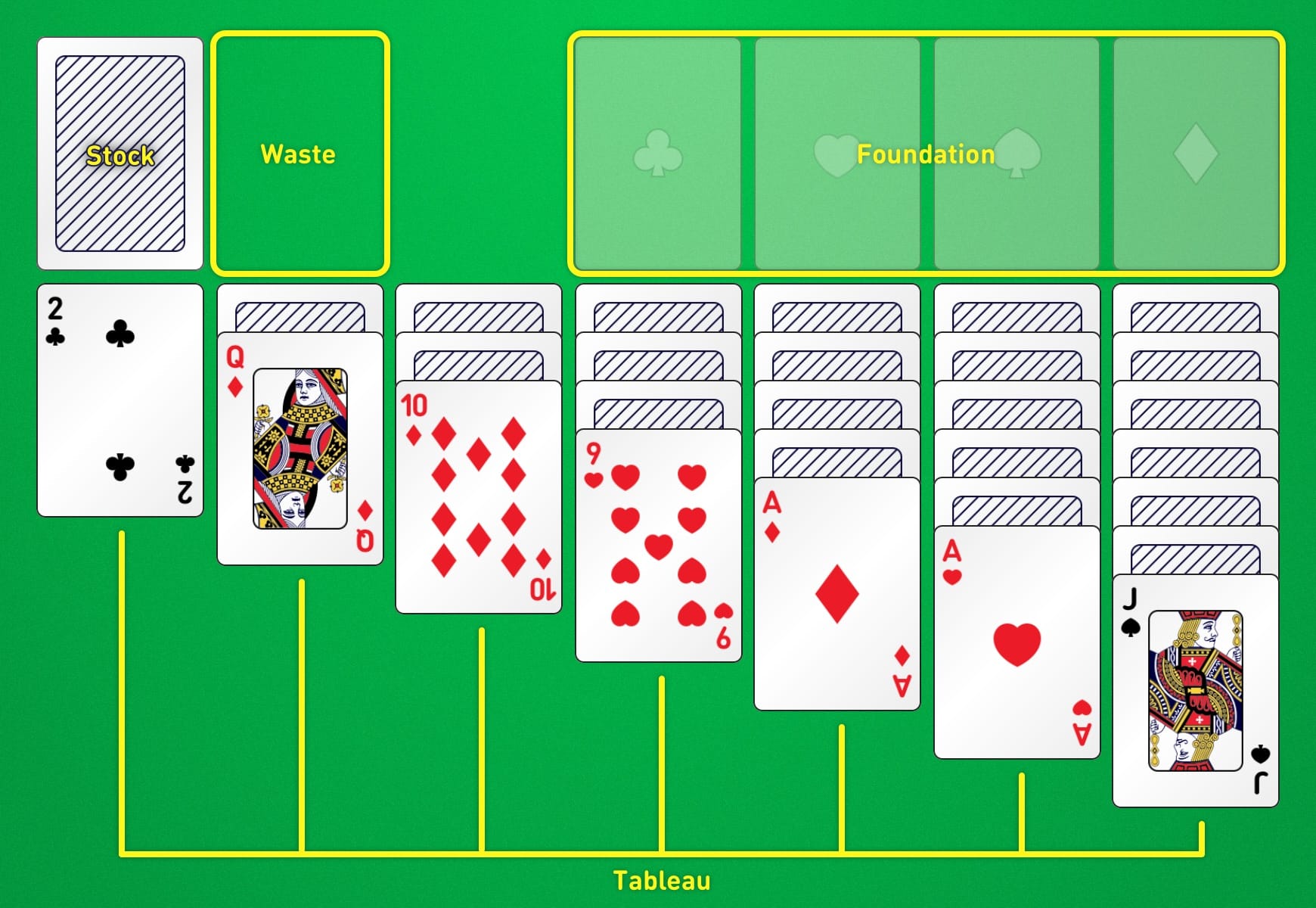
The whole process was a good reminder that you don’t need fancy tools or complex code to make something fun. Sometimes, the simplest ideas are the best.