Alright, let me tell you about wrestling with this “creator classic leaderboard” thing. It was a bit of a journey, not gonna lie.
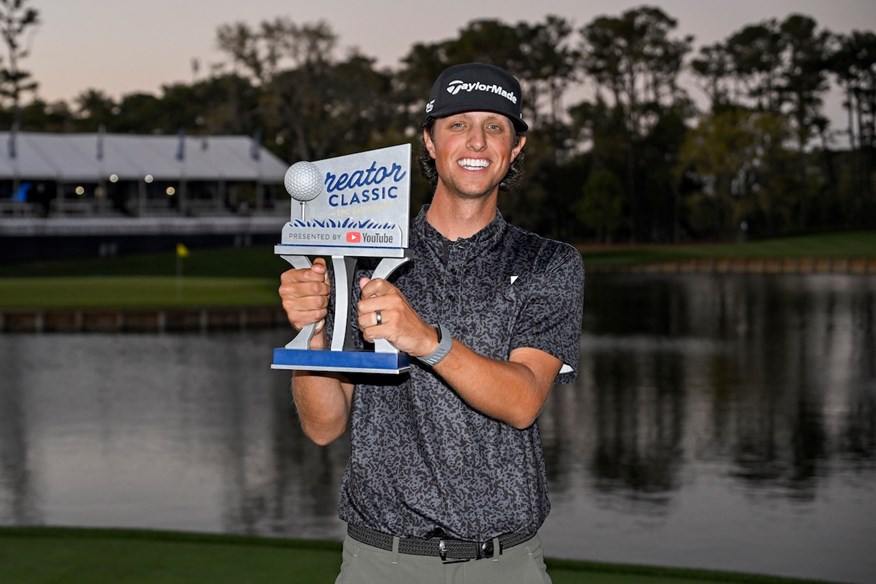
First off, I was tasked with creating a leaderboard system, you know, the kind that shows the top players in a game or app. Simple enough, right? Wrong!
I started by sketching out the basic structure. What data do I need? Player name, score, maybe a timestamp. So, I set up a basic data model. Nothing fancy, just plain old variables.
Then came the fun part – actually storing the data. I initially thought of using a simple array or list. Quick and easy, right? But then I realized I needed to sort it by score. So, I started looking into sorting algorithms. Man, that took me back to my college days!
I ended up using a simple bubble sort for the initial prototype. It’s not the fastest, but it’s easy to understand and implement. I figured I could optimize it later if needed.
Next, I needed a way to display the leaderboard. I created a UI element with a scrollable list. I dynamically populated the list with the data from my sorted array. It looked pretty basic, but it worked!
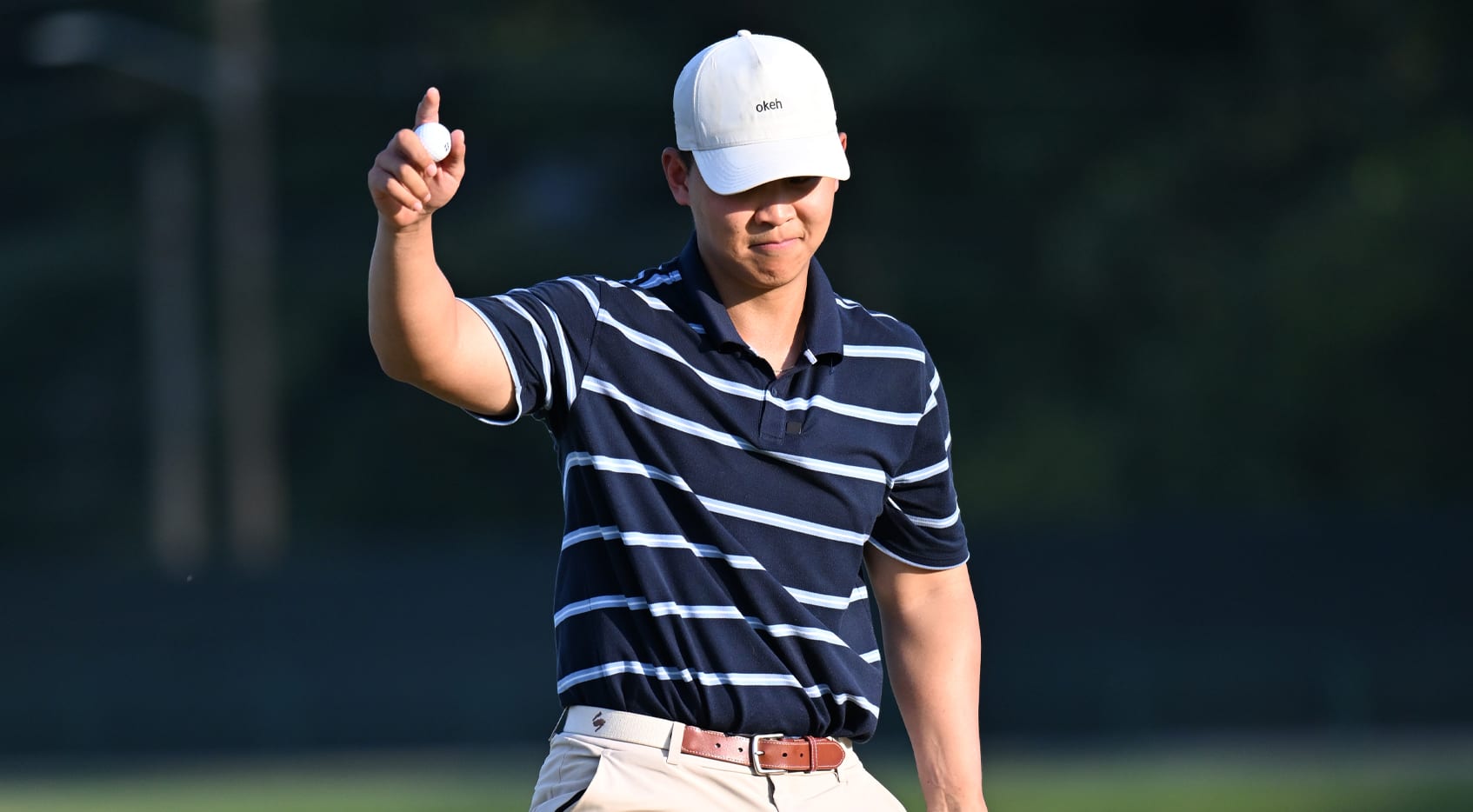
Now, here’s where things got interesting. I needed to persist the data. I didn’t want the leaderboard to reset every time the game restarted. So, I looked into saving the data to a file. I ended up using JSON. It’s human-readable and easy to work with.
I wrote functions to load and save the leaderboard data to a JSON file. Every time the game ended, the leaderboard was updated and saved. Every time the game started, the leaderboard was loaded from the file.
But the leaderboard felt static. I decided to add some visual flair. I highlighted the player’s own score in the leaderboard. I also added some animations to the list items.
To make it more engaging, I added a feature where players could submit their score to the leaderboard. I created a simple input field and a submit button. When the player submitted their score, it was added to the leaderboard and saved.
I also added some error handling. What if the player entered an invalid score? What if the save file was corrupted? I added checks and error messages to handle these situations.
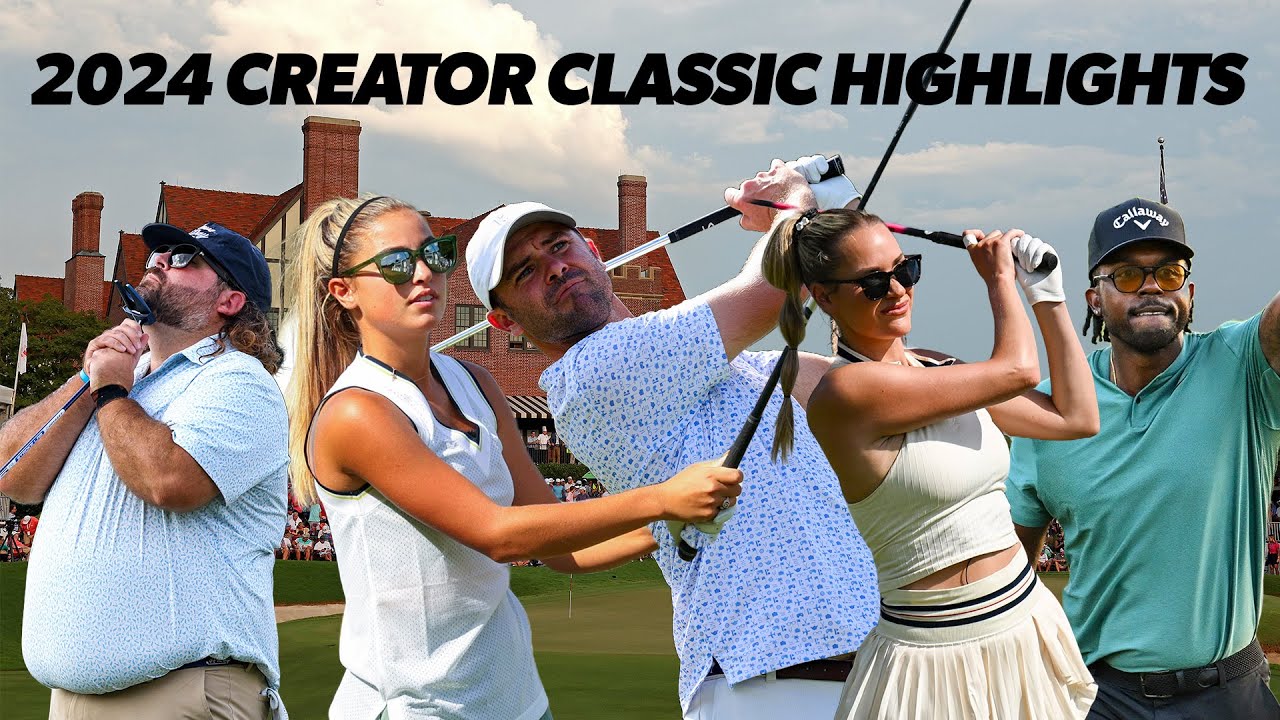
Finally, I tested the leaderboard thoroughly. I played the game multiple times, trying different scores and scenarios. I also asked my friends to test it and give me feedback.
After a few iterations of testing and bug fixing, the leaderboard was finally working smoothly. It was a challenging but rewarding experience. I learned a lot about data structures, algorithms, and persistence.
Here’s the gist of what I did:
- Created a data model for player scores.
- Used a bubble sort algorithm to sort the leaderboard.
- Displayed the leaderboard in a UI scrollable list.
- Persisted the leaderboard data using JSON.
- Added visual flair and animations.
- Implemented score submission functionality.
- Added error handling and validation.
- Tested the leaderboard thoroughly.
It was a real grind, but seeing that leaderboard in action made it all worth it!