Okay, so here’s the deal. Today, I’m diving into my experience with building a “marcus smart contract.” It was a bit of a rollercoaster, so buckle up!
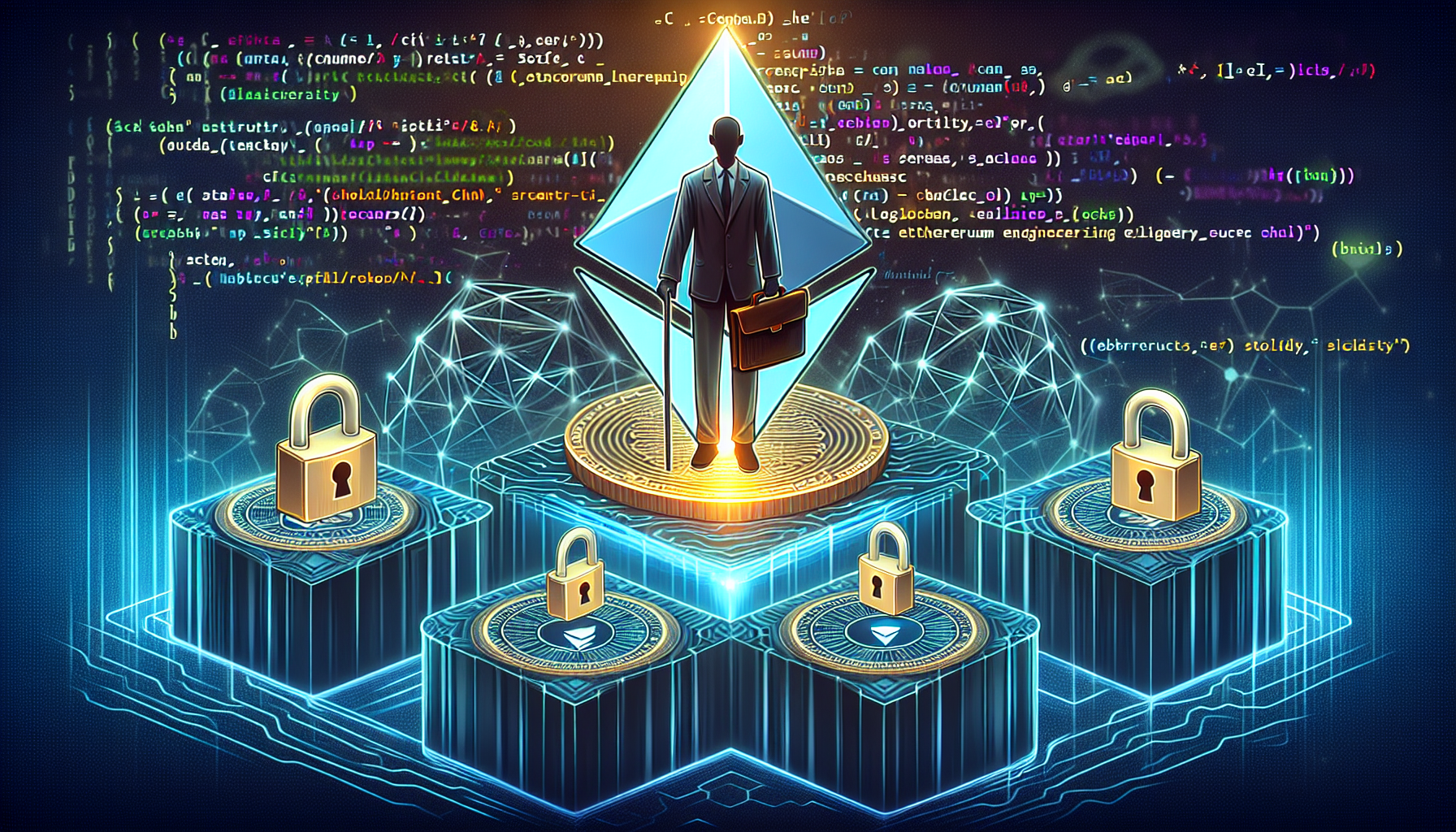
First off, I had this idea bouncing around in my head. I wanted to create a simple smart contract. I figured, how hard could it be, right? Famous last words.
So, I jumped straight into Remix, the online Solidity IDE. It’s pretty handy for quick prototyping. I started sketching out the basic structure of my contract. I wanted it to have a function to set a value, and another function to read that value. Classic stuff.
I started by declaring the state variable that was going to hold my data. Something super simple, like a uint256
called myValue
. Then, I wrote the setValue
function. This was supposed to take a uint256
as input and update myValue
.
solidity
pragma solidity ^0.8.0;
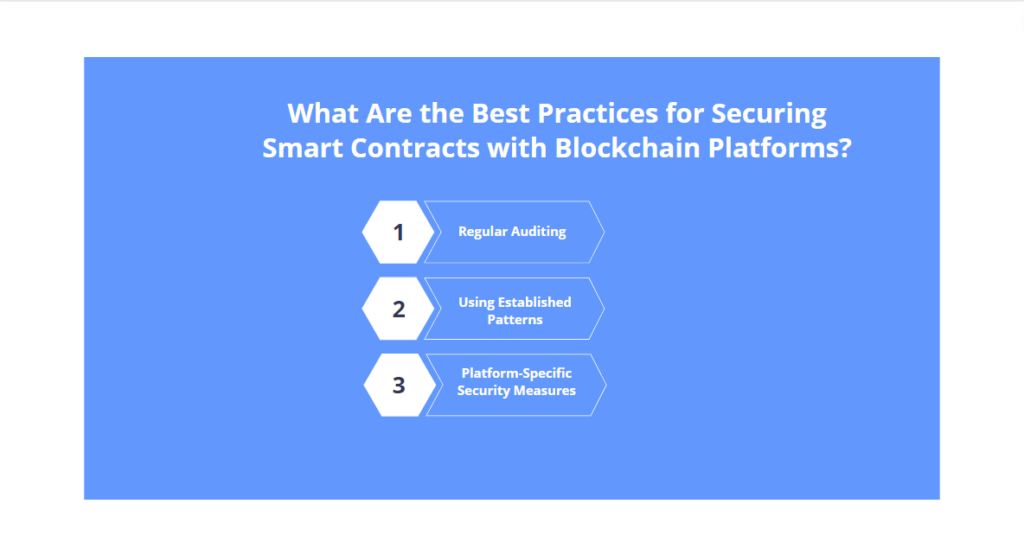
contract MarcusContract {
uint256 public myValue;
function setValue(uint256 _newValue) public {
myValue = _newValue;
function getValue() public view returns (uint256) {
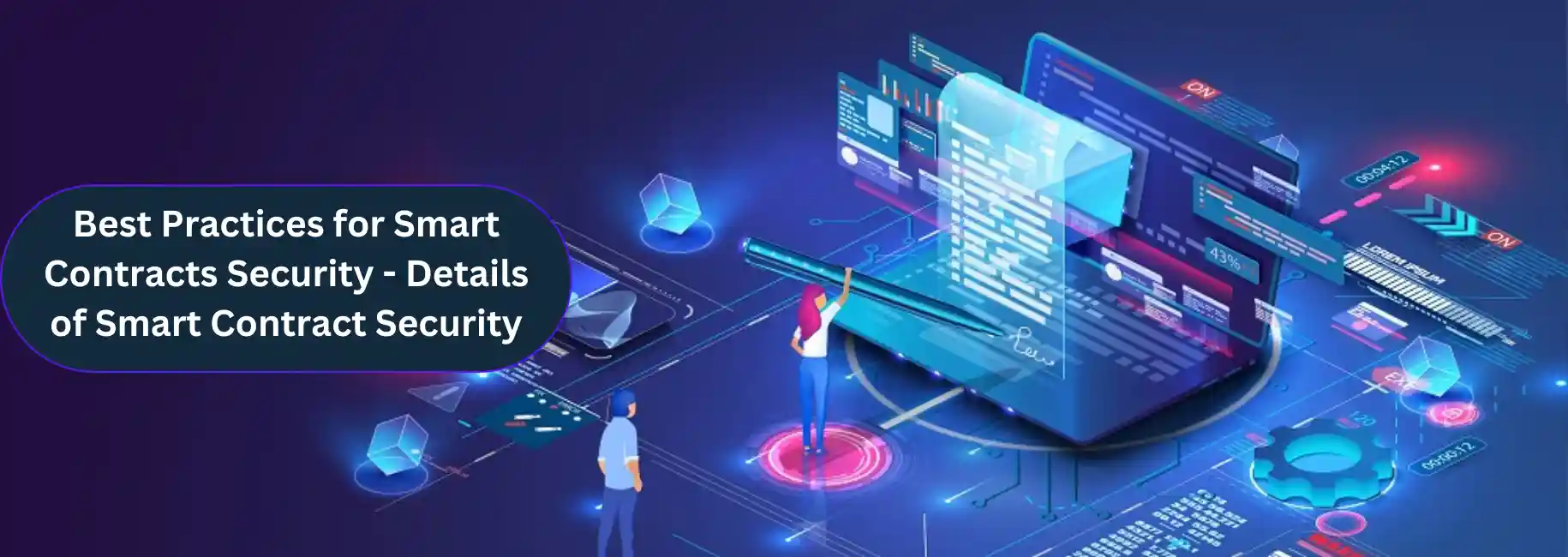
return myValue;
I deployed it to the Remix VM. Tried calling the setValue
function with different numbers. Checked if the getValue
function returned the correct value. Everything seemed fine and dandy.
Next, I decided to get a little fancy. I wanted to add an event that would be emitted whenever the value was changed. Just for the heck of it. I added an event declaration and an emit
statement inside the setValue
function.
solidity
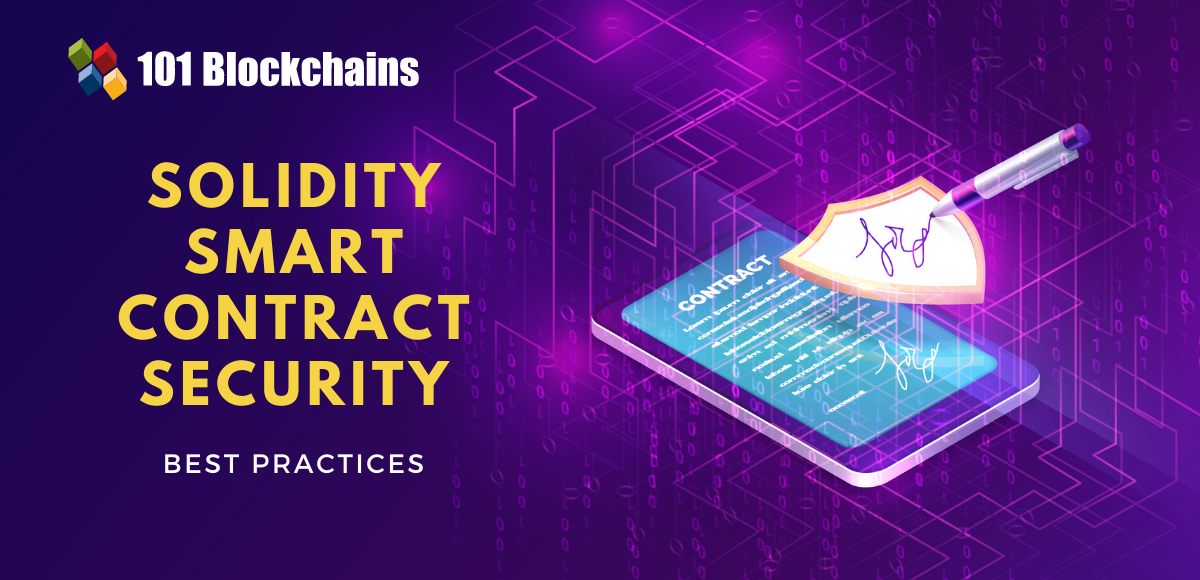
pragma solidity ^0.8.0;
contract MarcusContract {
uint256 public myValue;
event ValueChanged(uint256 newValue);
function setValue(uint256 _newValue) public {
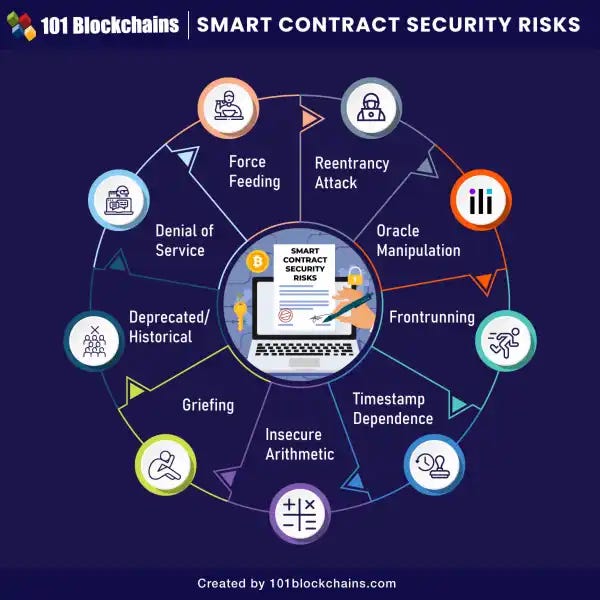
myValue = _newValue;
emit ValueChanged(_newValue);
function getValue() public view returns (uint256) {
return myValue;
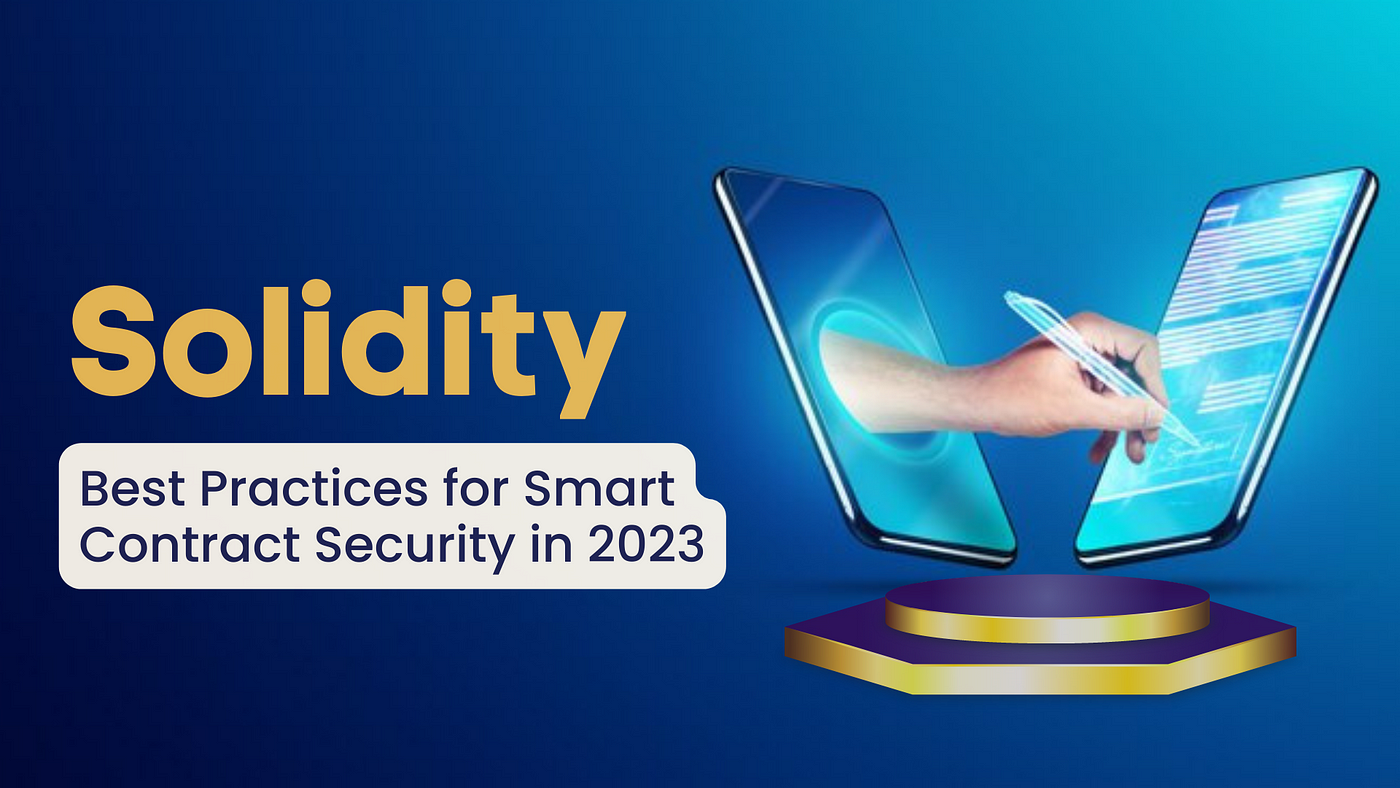
Redeployed, tested again. Yep, events were firing as expected. I patted myself on the back at this point.
Then I thought about security. I mean, anyone could call setValue
and change the value. That’s no good. I wanted only the contract creator to be able to modify it.
So, I added a constructor
to store the address of the deployer and a require
statement in the setValue
function to check if the caller is the owner.
solidity
pragma solidity ^0.8.0;
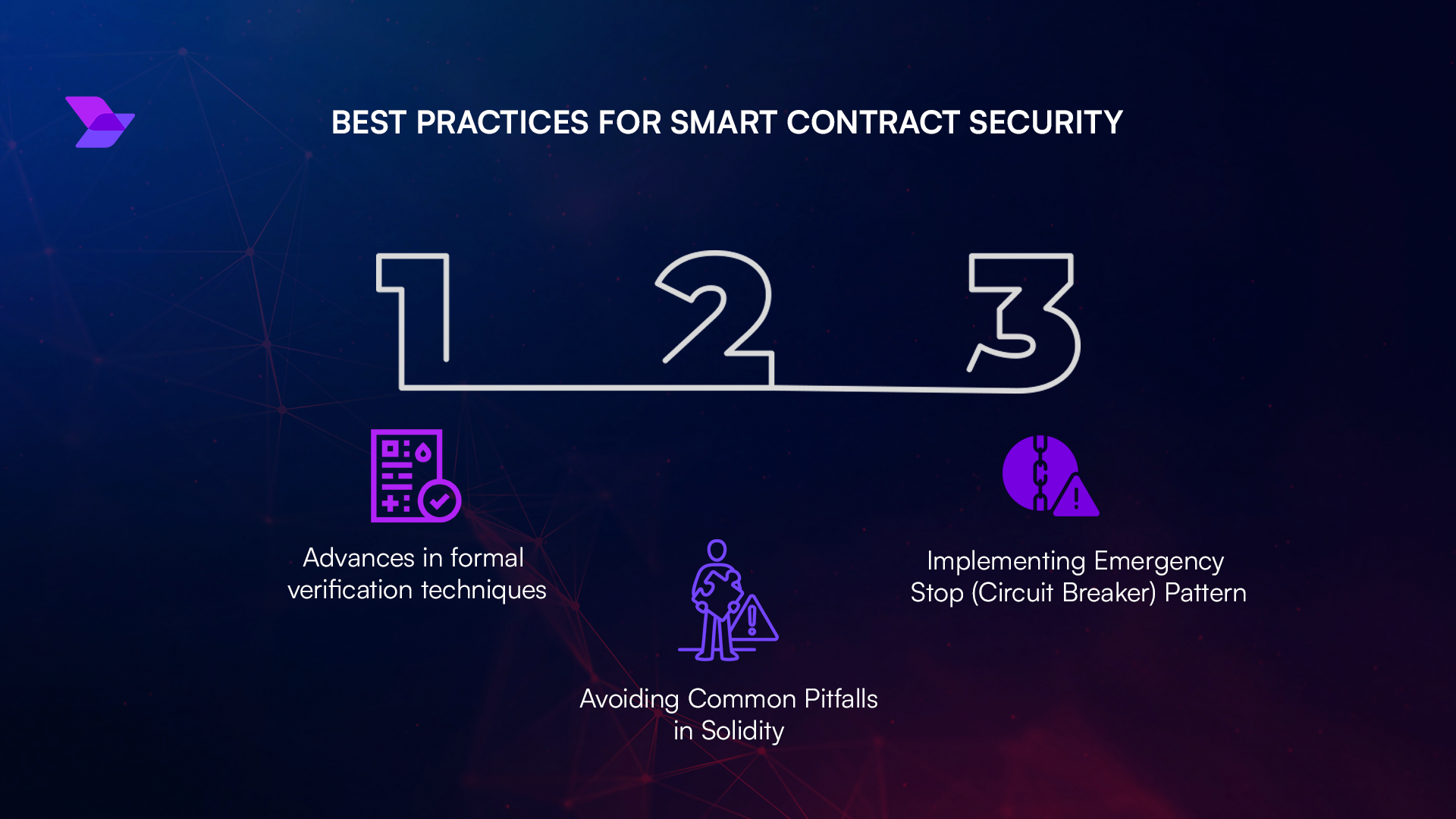
contract MarcusContract {
uint256 public myValue;
address public owner;
event ValueChanged(uint256 newValue);
constructor() {
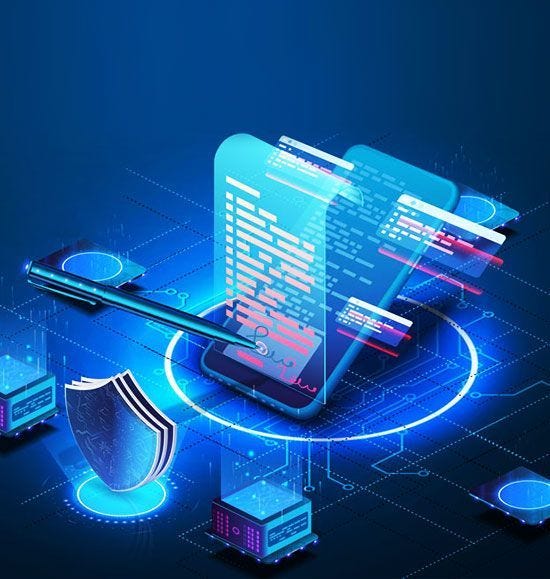
owner = *;
function setValue(uint256 _newValue) public {
require(* == owner, “Only the owner can set the value.”);
myValue = _newValue;
emit ValueChanged(_newValue);
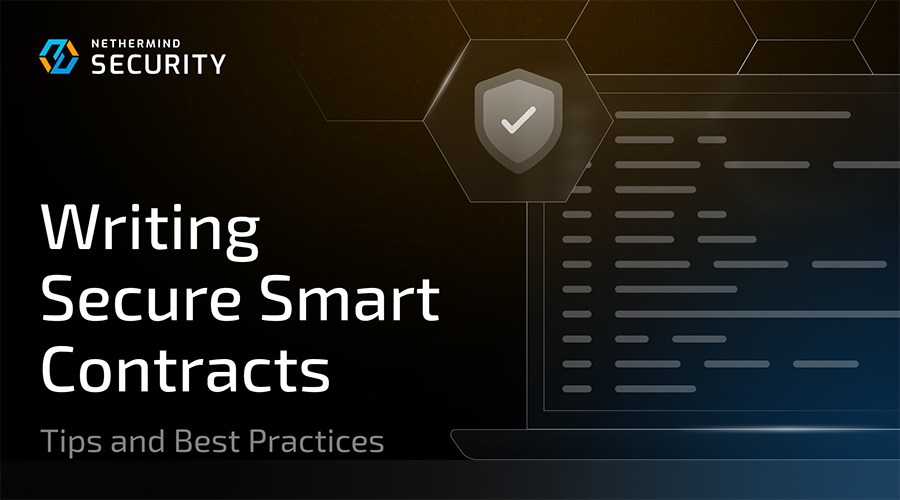
function getValue() public view returns (uint256) {
return myValue;
Deployed it to a testnet, tried calling setValue
from a different account. BAM! “Only the owner can set the value.” message. Success!
Now, for the final touch, I wanted to make the contract a bit more… interesting. I decided to add a function that would multiply myValue
by a given factor.
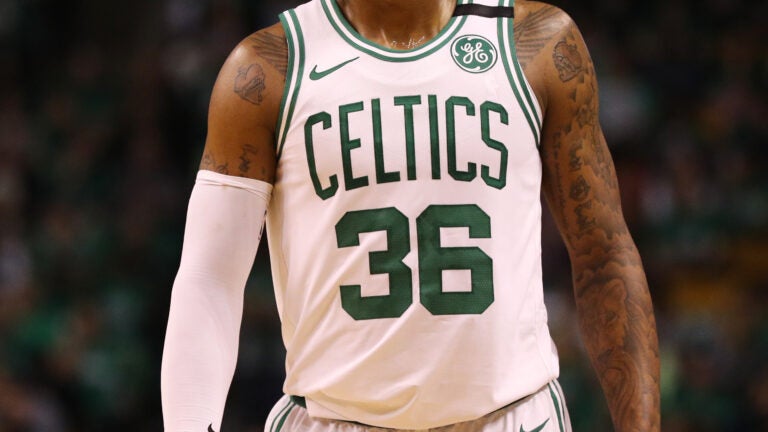
solidity
pragma solidity ^0.8.0;
contract MarcusContract {
uint256 public myValue;
address public owner;
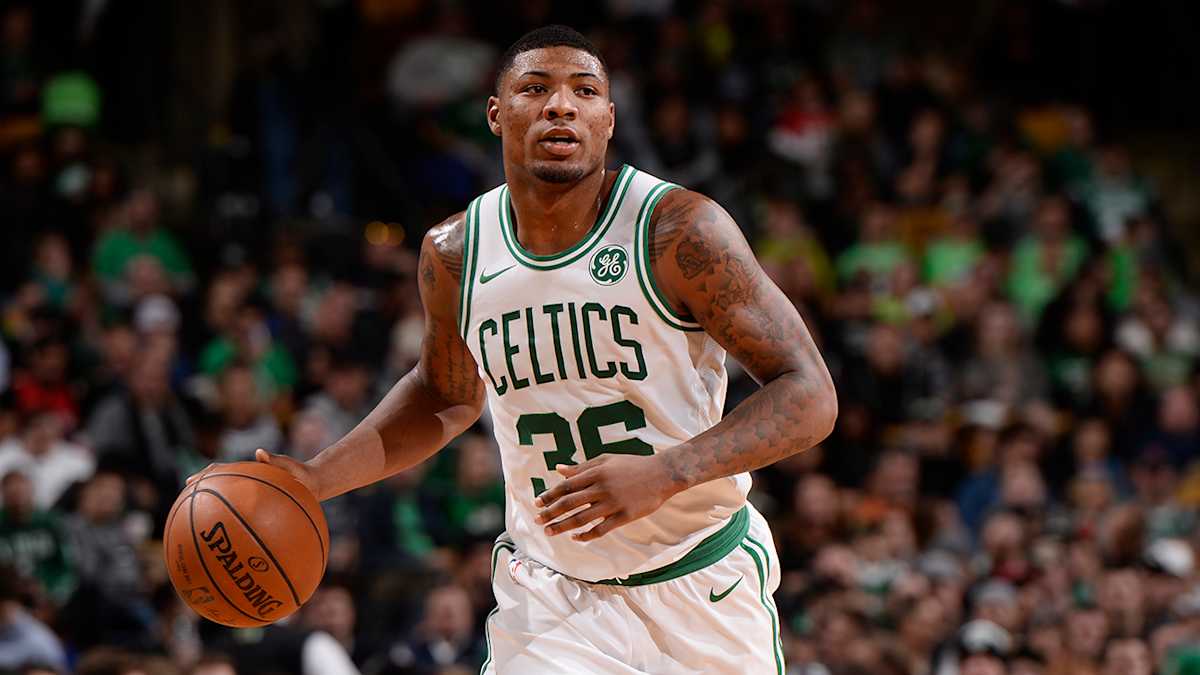
event ValueChanged(uint256 newValue);
constructor() {
owner = *;
function setValue(uint256 _newValue) public {
require(* == owner, “Only the owner can set the value.”);
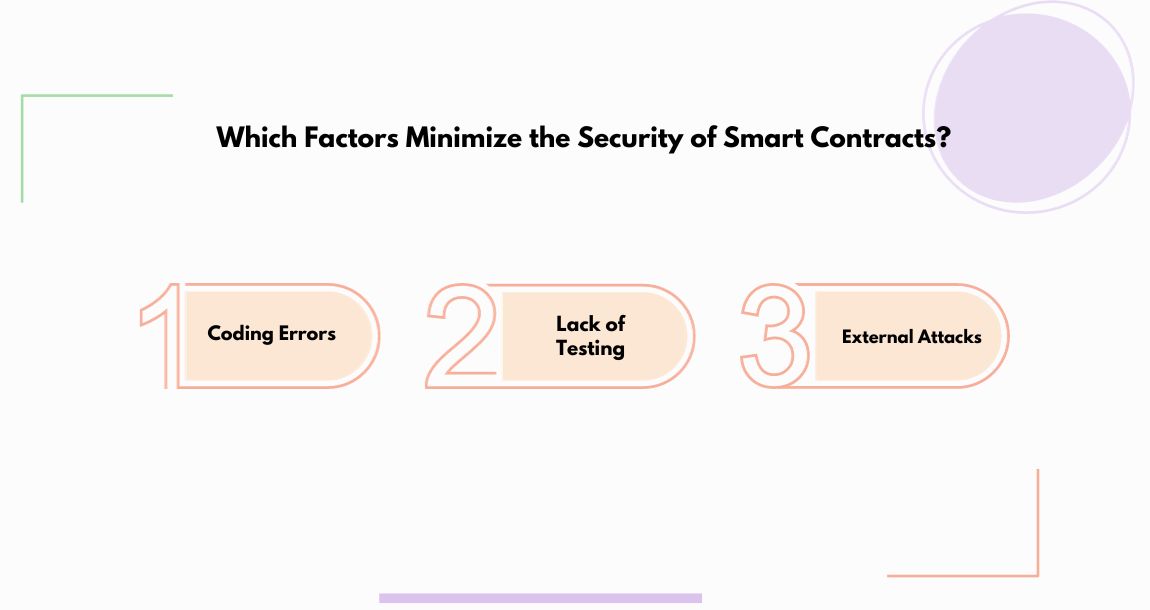
myValue = _newValue;
emit ValueChanged(_newValue);
function getValue() public view returns (uint256) {
return myValue;
function multiplyValue(uint256 _factor) public {
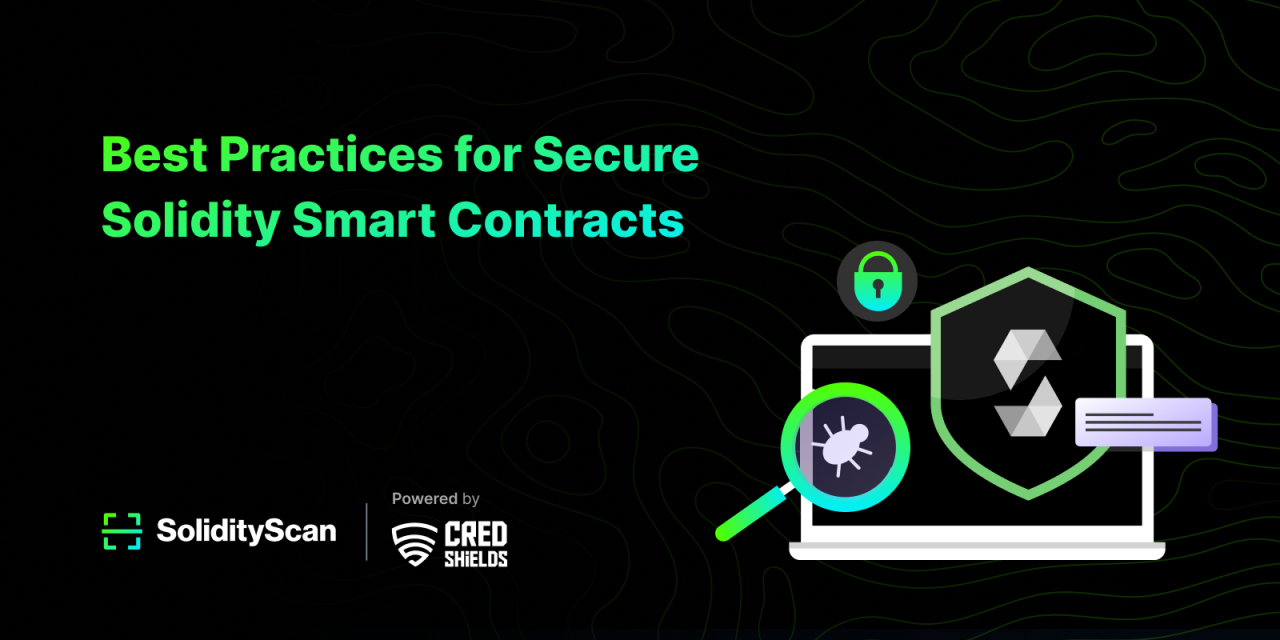
require(* == owner, “Only the owner can multiply the value.”);
myValue = myValue _factor;
emit ValueChanged(myValue);
Deployed it again. Tried multiplying the value by different numbers. Worked like a charm. It was cool seeing the value change and the events firing.
It wasn’t super complex, but it was a great exercise. I touched on basic state variables, functions, events, modifiers, and even a little bit of security. It’s all about taking small steps and building from there.