Okay, so today I decided to tackle a fun little project – building a solver for the Blossom Puzzle. You know, that flower-shaped puzzle where you have to fit all the pieces together perfectly? I’ve always loved puzzles, and I figured this would be a good way to kill some time and maybe learn something new.
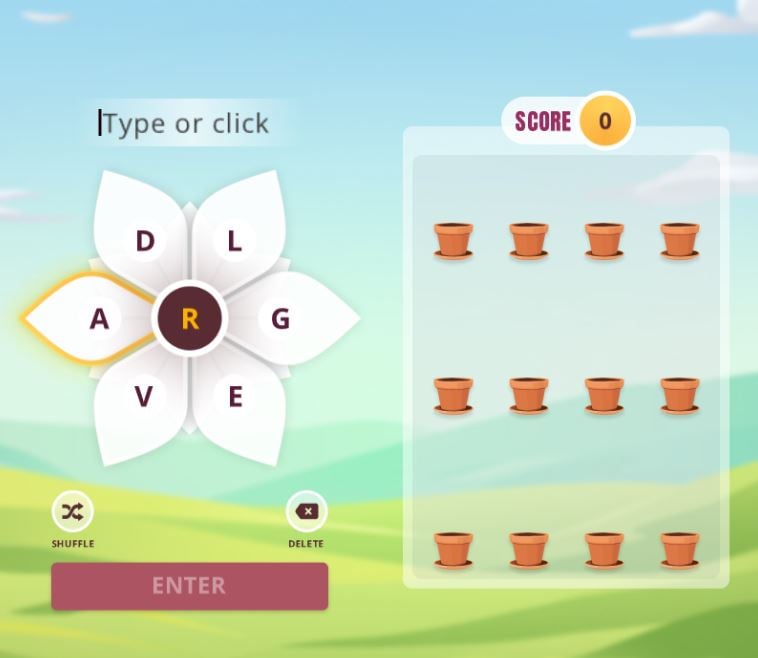
Getting Started
First, I grabbed the puzzle itself. Mine is a physical, wooden one, but I guess you could do this with a digital version too. I spent a good amount of time just playing with it, trying to get a feel for how the pieces interact. This is important, I think, to understand the underlying ‘rules’ before you try to automate anything.
Figuring Out the Logic
Next, I started thinking about how to represent the puzzle in a way a computer could understand. I figured I could probably use some kind of grid system, with each cell representing a possible location for a piece. The pieces themselves could be represented by their shape and orientation.
- Shapes: I noticed there were only a few basic shapes, even though some were rotated versions of others.
- Orientation: This was key! Each piece could potentially fit in multiple orientations.
Trying Things Out (and Failing a Lot!)
I started by trying a really simple, brute-force approach. Basically, I wrote some code to try placing each piece, one by one, in every possible location and orientation. If it fit, great! Move on to the next piece. If not, try the next spot, or the next rotation, and so on.
It… didn’t work very well, at least not at first. My code would get stuck a lot, going down these rabbit holes of placements that clearly weren’t going to work. It was like watching someone try to solve a jigsaw puzzle by randomly jamming pieces together.
Making It Smarter
I realized I needed to be a bit more clever. So I added some checks to my code. Things like:
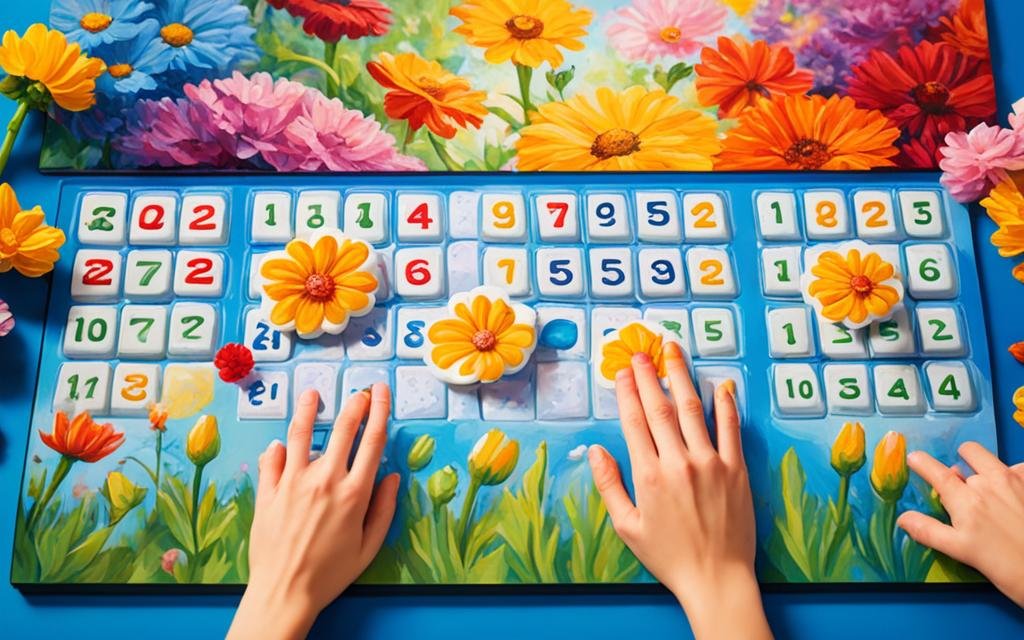
- Edge detection: If a piece was hanging off the edge of the puzzle, don’t even bother trying to place it.
- Overlap checking: Make sure a piece isn’t overlapping with any previously placed pieces.
These changes helped a lot. My solver was still pretty slow, but it was at least making some progress, and fewer obviously wrong moves.
More Refinements (and More Coffee)
I kept tweaking things. I added a “backtracking” feature, so if the solver got stuck, it could go back and try a different arrangement of earlier pieces. This was a game-changer! It still took a while, but it actually started solving the puzzle sometimes!
Finally Solved It
It took a few more hours of fiddling, debugging, and drinking way too much coffee, but I finally got it working! My solver could now reliably solve my Blossom Puzzle. It’s not the fastest solver in the world, and I’m sure there are tons of ways to optimize it, but it works, and that’s what matters.
This was a really satisfying project. It wasn’t easy, but it was fun to wrestle with the problem and slowly chip away at it until I had a working solution. It’s a good reminder that even seemingly simple problems can have surprising depth, and that perseverance pays off. Plus, now I have a cool program that can solve my puzzle for me!