Okay, so I wanted to mess around with some scripting, and I had this idea for something I’m calling “celtics script”. It’s not related to basketball. Don’t ask about the name, that’s just what popped into my head. Basically, I wanted to see if I could automate a thing, using python, that I was doing manually before. I wanted to make a script that takes a file full of separate words and then makes a new file with those same words, just combined in lines of five words.
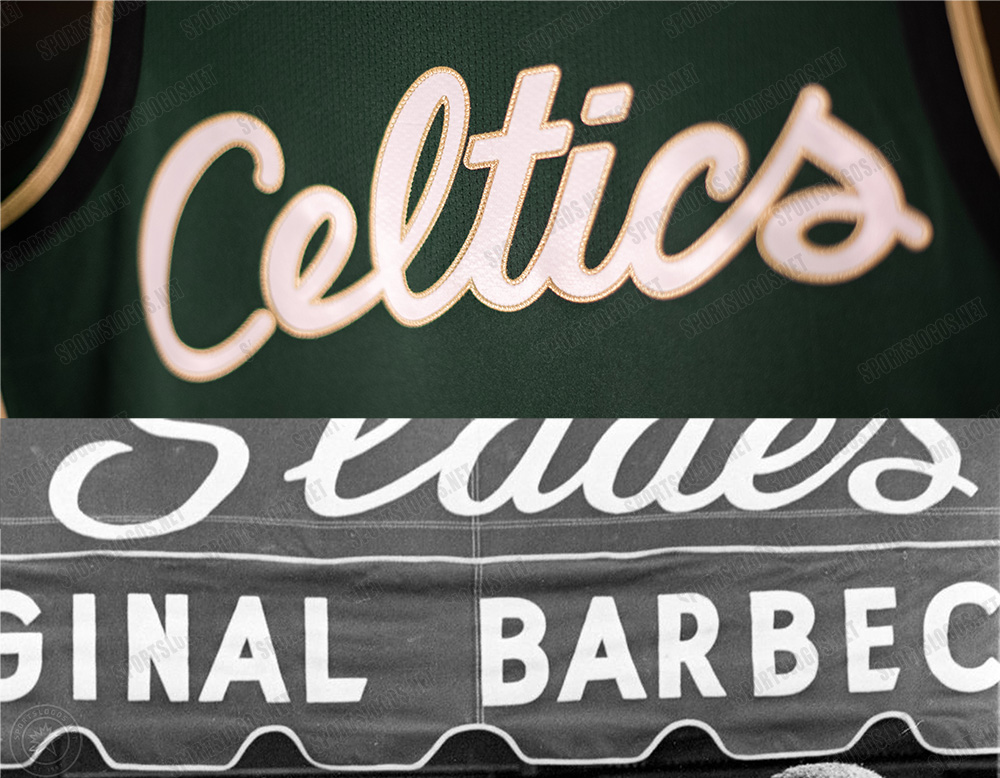
Getting Started
First, I made a new folder on my desktop. Just to keep things tidy. I called it, cleverly, “celtics_script_project”. Inside that, I created two empty text files: “*” and “*”. I opened up “*” and just started typing a bunch of random words, each on its own line. Like, “apple”, “banana”, “computer”, “dog”, “elephant”, you get the idea. I filled up, like, two pages worth.
Then I opened up my trusty code editor. I’m using a basic one. Time to write some Python!
The Script Itself
I started by importing the `os` module, it is powerful. I figured I’d need that for working with files.
I started with simple code as below.
python
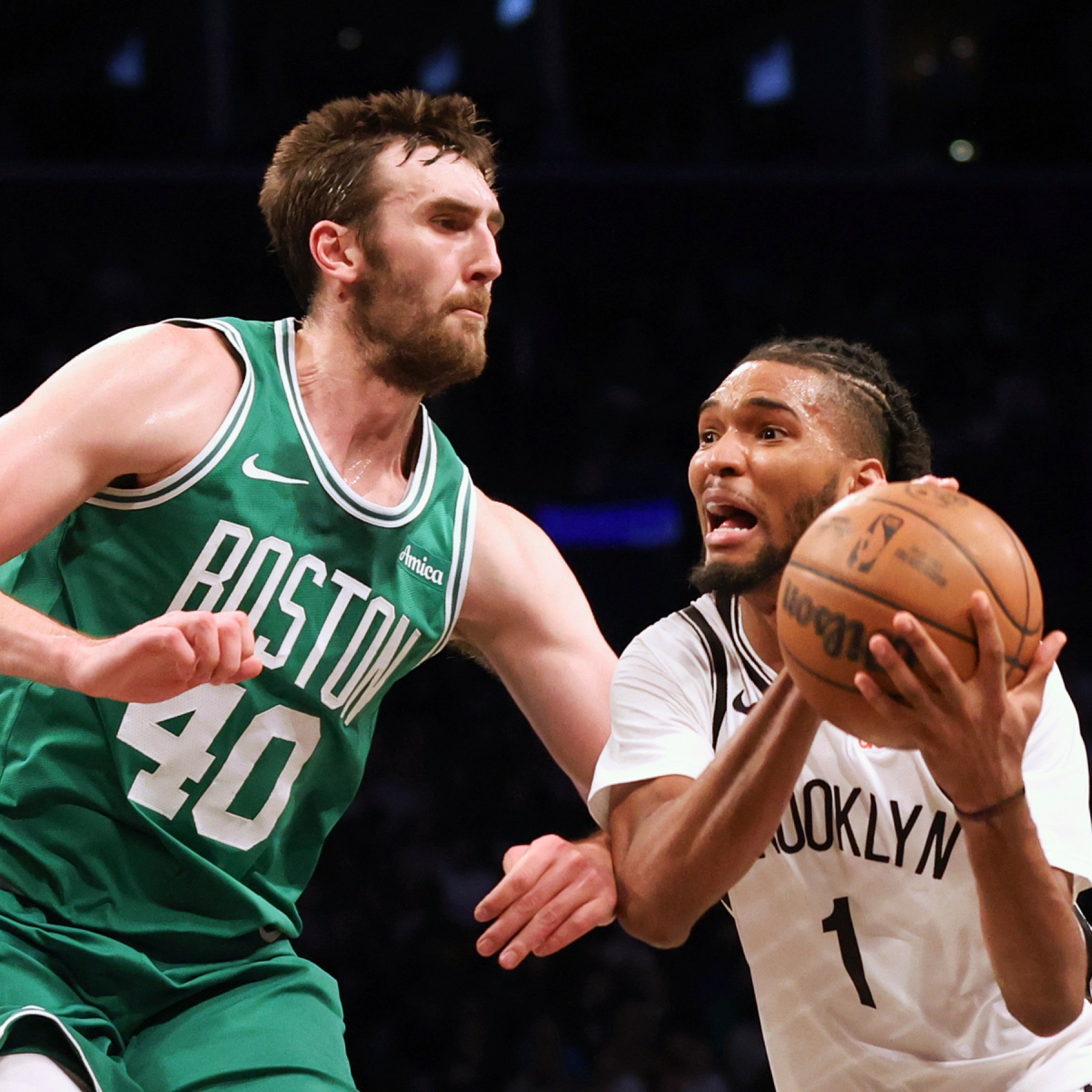
import os
Then, I defined the file paths. I did it using relative paths because the files are in the same directory as the script. Less typing that way.
python
input_file = “*”
output_file = “*”
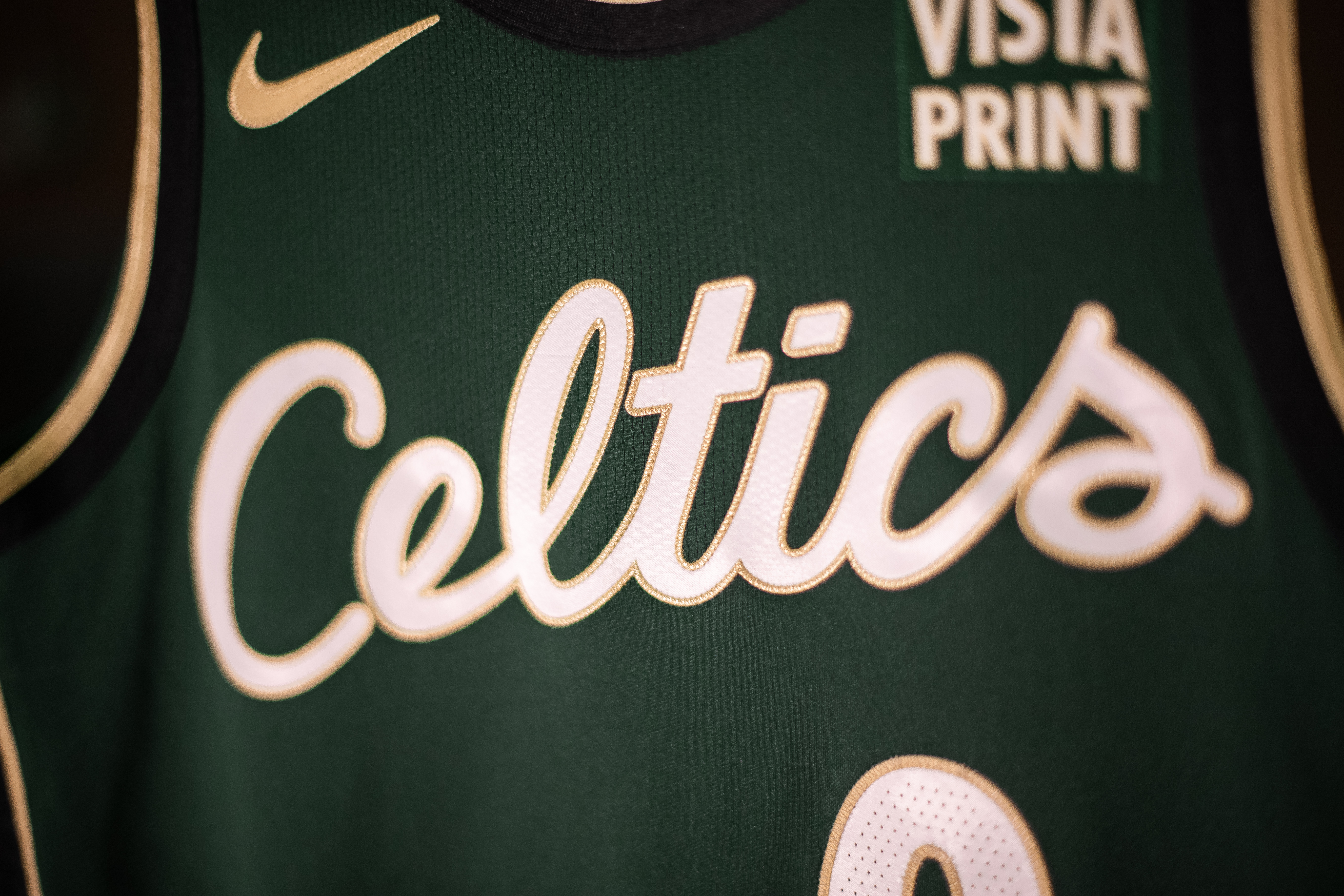
I used a simple `with open()`. The basic stuff. I opened the “*” file in read mode (“r”) and the “*” file in write mode (“w”).
python
with open(input_file, ‘r’) as infile, open(output_file, ‘w’) as outfile:
# rest of the code will go inside this block
Now for the main part. I created an empty list called `word_list`. Then, I looped through each line in the input file. For each line, I used `.strip()` to get rid of any leading or trailing whitespace (like extra spaces or newlines). Then I used `.split()` to, well, split the line into individual words (in case there was more than one word on a line, which there shouldn’t have been, but better safe than sorry). I then extended my `word_list` with these words.
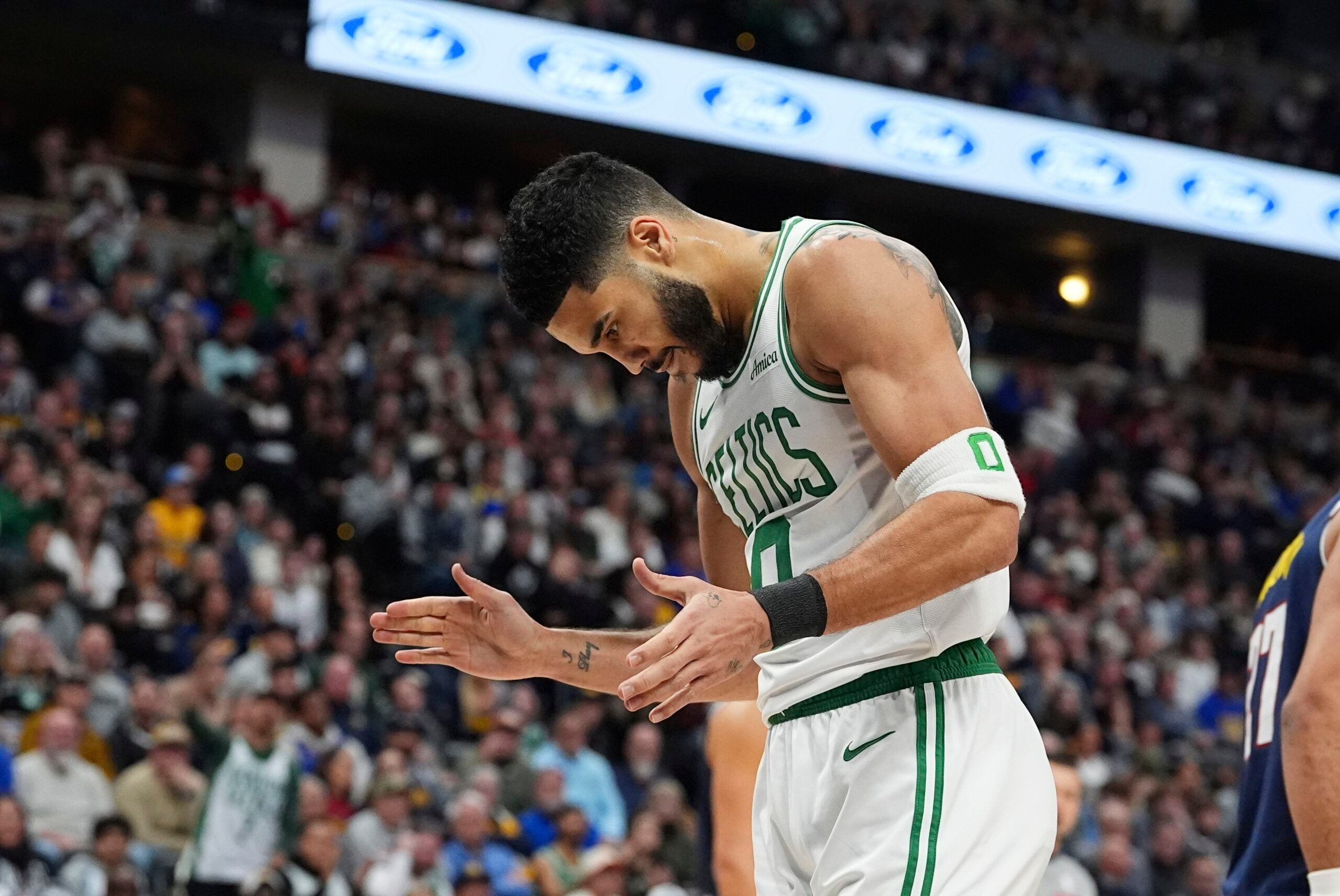
python
word_list = []
for line in infile:
words = *().split()
word_*(words)
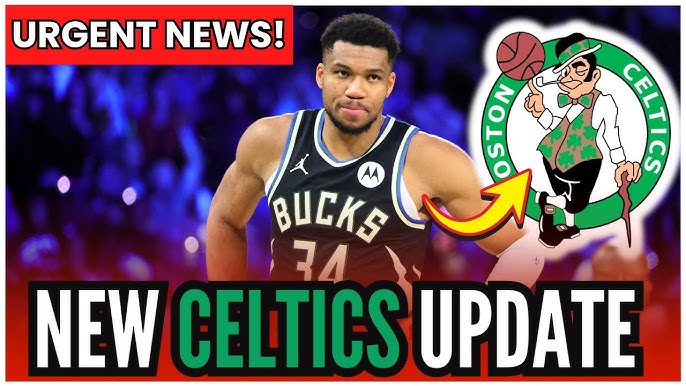
The core logic! I used a `for` loop and `range()` to iterate through the `word_list` in chunks of five. The `range(0, len(word_list), 5)` part is the key here. It starts at index 0, goes up to the length of the `word_list`, and increments by 5 each time.
python
for i in range(0, len(word_list), 5):
# get a chunk of 5 words
chunk = word_list[i:i+5]
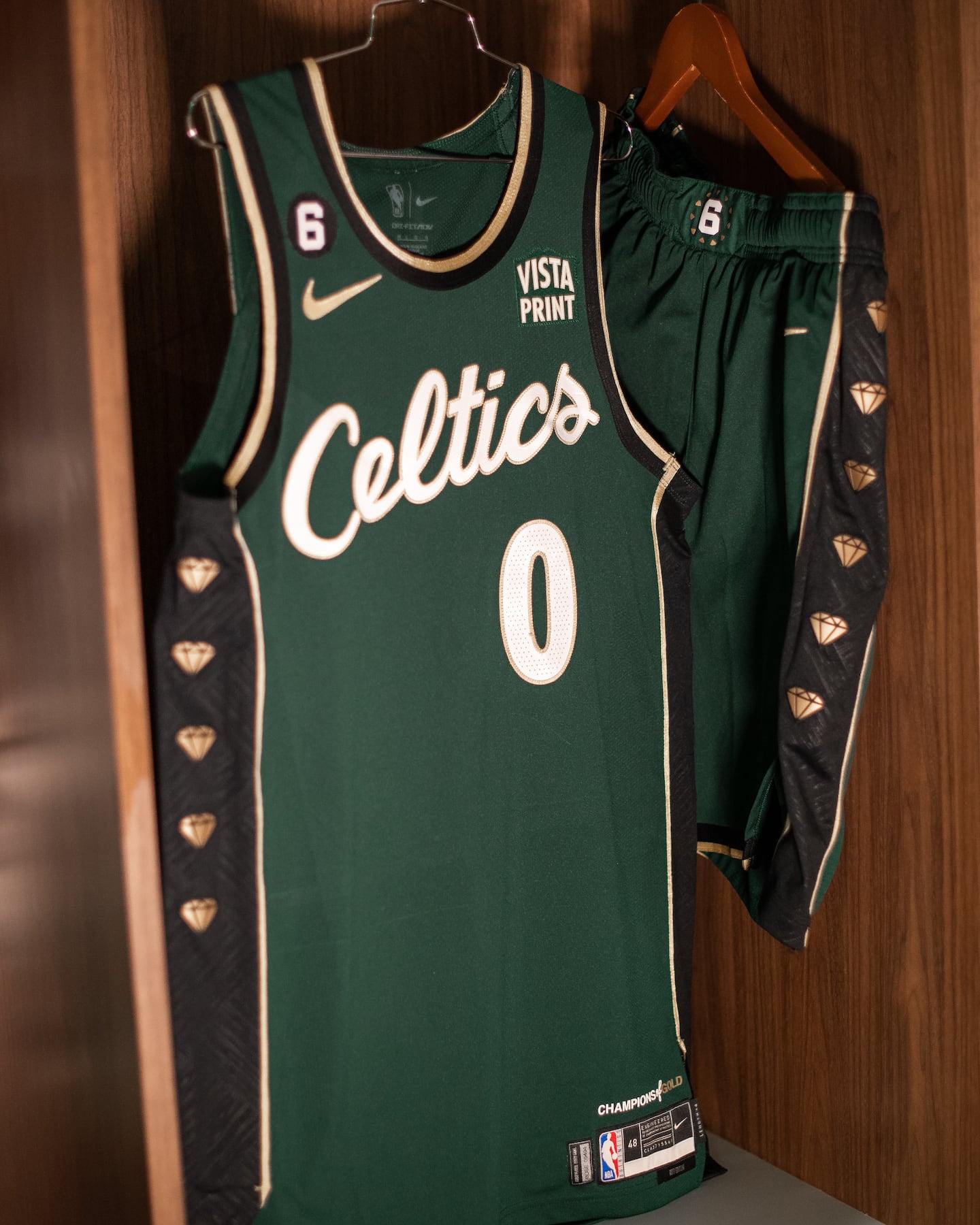
Inside the loop, `word_list[i:i+5]` grabs a “slice” of the list, from index `i` up to (but not including) `i+5`. So, it gets five words at a time (or fewer, if we’re at the end of the list and there aren’t five words left). Then I use `’ ‘.join(chunk)` to join those words together, separated by a single space. And finally, I write that joined string to the output file, followed by a newline character (`n`) to put each group of five words on its own line.
python
*(‘ ‘.join(chunk) + ‘n’)
Running and Testing
I saved the script as “*” in the same folder as my text files. I opened up a terminal (or command prompt), navigated to that folder using `cd`, and then ran the script with `python *`.
I opened up “*” and… there it was! All my random words, neatly arranged in groups of five on each line. It worked! I felt a little surge of accomplishment. I mean, it’s a simple script, but it did what I wanted it to do.
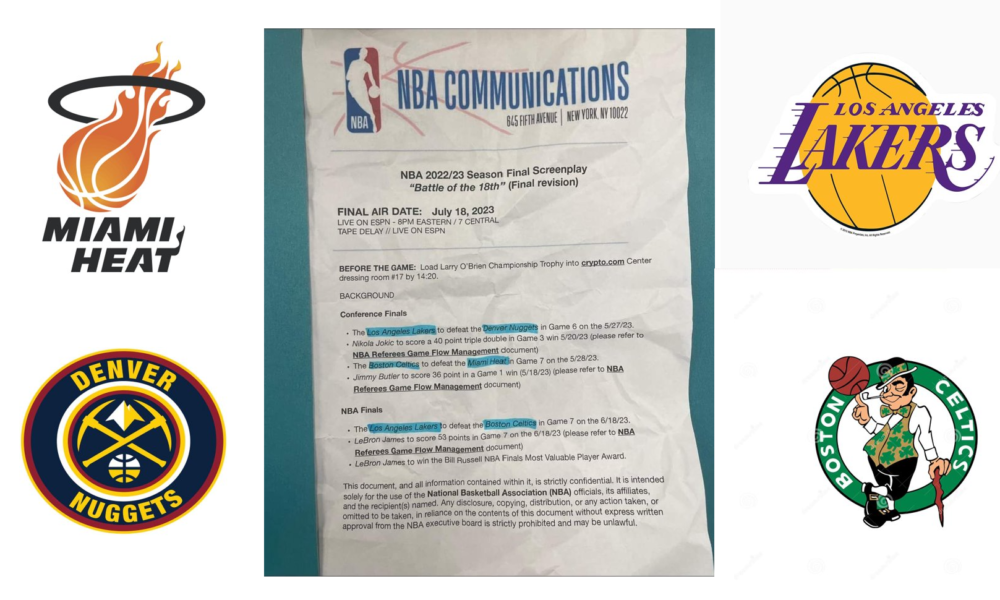
I then played around with it some more. I tried adding more words to “*”, running the script again, and checking “*”. Perfect. I even tried putting some extra spaces and blank lines in “*” to see if my `.strip()` was working correctly, and it was. All handled nice and clean.
The whole process, from start to finish, probably only took me, like, less than half an hour, including writing this up. That’s the beauty of these little scripting projects. You can automate a task, learn something new, and get that satisfying feeling of making the computer do your bidding, all in a relatively short amount of time. Now I’ve got this handy little script I can use whenever I need to do this kind of word-grouping thing.