Okay, so I’ve been wanting to mess around with Jersey and JSON, and I figured a good way to do it would be to try and whip up a simple REST endpoint. I’m a huge basketball fan, and Jason Kidd is a legend, so I thought, “Why not combine these things?” Here’s how I went about it, bumps and all.
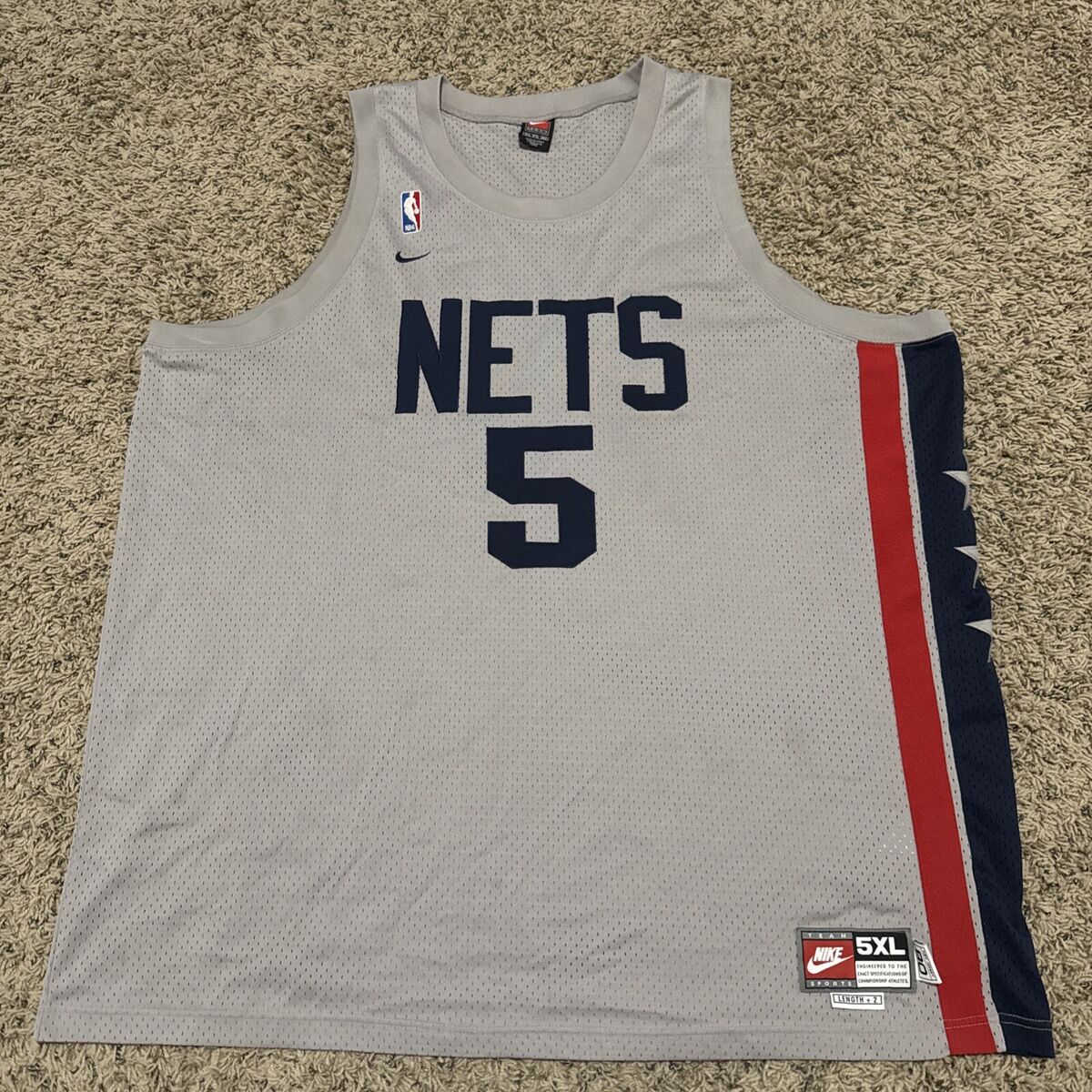
Setting Up the Project
First things first, I needed a new project. I fired up my IDE and created a new Maven project. I made sure to check that “Create a simple project (skip archetype selection)” box, ’cause I like to keep things… well, simple, at least at the start.
Next up, the *. This is where I added all the stuff I needed. I grabbed the latest Jersey dependencies – the core server, the client, and the JSON support. I went with the ‘jersey-media-json-jackson’ for the JSON processing since that’s seemed pretty common in the examples I’d seen.
Coding the Resource
With the project set up, I started coding. I made a new Java class, called it “KiddResource”. This is where the magic (or at least, the endpoint) would live. I slapped a @Path("/kidd")
annotation on the class. This basically tells Jersey, “Hey, when someone hits /kidd, this is the class to handle it.”
Then, I created a method to actually get some Kidd data. I called it, imaginatively, getKidd()
. I threw a @GET
annotation on it, and a @Produces(*_JSON)
to tell Jersey this method returns JSON.
Inside the method, I initially just created a simple String, something like “{“name”: “Jason Kidd”, “team”: “Nets”}”. I figured I’d start simple and build from there. I returned this String.
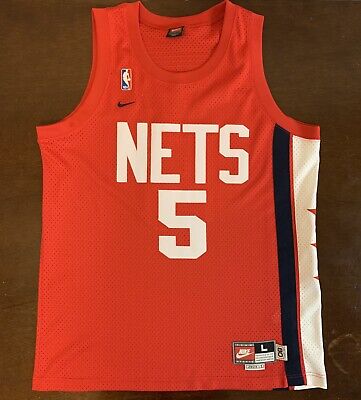
Running and Testing
Time to see if this thing actually worked. I deployed the application to a local server (I use Tomcat, but you do you). Then, I opened up my browser and typed in the URL: something to the order of ‘…/my-app/kidd’.
And… boom! There it was, my JSON string, staring back at me. “Not bad,” I thought, “but pretty basic.”
Adding Some Real Data
Next step, make it a little more interesting. I decided to create a simple “Player” class, with fields like “name,” “team,” “position,” and maybe “jerseyNumber.” I populated a Player
object with some Jason Kidd stats (gotta represent those Nets years!).
Then, I went back to my getKidd()
method. Instead of returning a hardcoded String, I returned the Player
object. I figured Jersey and Jackson would handle the conversion to JSON for me. And they did!
- Refreshed the page, and the JSON output looked great.
- Much better than my hardcoded string, with all fields properly labeled.
Experimenting with Different Responses
Just for kicks, I created another method in my KiddResource
, this time with a @POST
annotation. I wanted to see if I could send some data to the server. This one was a little trickier, because I had to figure out how to handle the incoming JSON data.
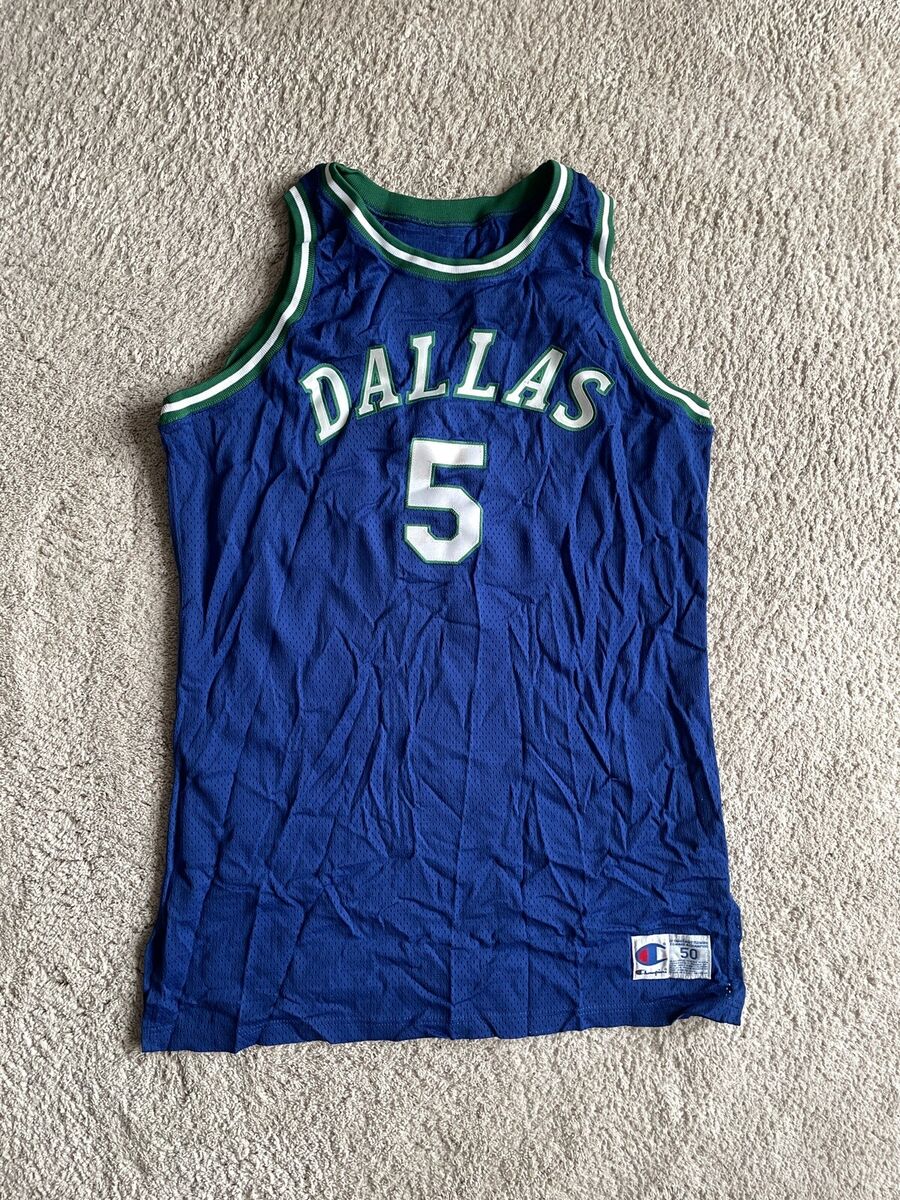
I used the @Consumes(*_JSON)
annotation to tell Jersey this method expected JSON. In the method body, I used Jackson’s ObjectMapper
to convert the incoming JSON string into a Player
object. For now, I just printed the object’s details to the console, just to confirm it was working.
I used a REST client (Postman is my go-to) to send a POST request with some JSON data. Checked the console, and sure enough, the Player
object was created correctly. Success!
Wrapping Up
So, that’s basically it. I got a simple Jersey endpoint up and running, serving up some Jason Kidd data in JSON format. I played around with both GET and POST requests, and got a feel for how Jersey handles JSON with Jackson. It’s not rocket science, but it’s a solid start. Next, I will probably try connecting it to a real database, so I’m not just pulling data out of thin air. But for now, I’m calling it a win.